From hexadecimal to one's complement in Python
13,210
Solution 1
Just use the XOR operator ^
against 0xFF:
>>> hex(0x9E ^ 0xFF)
'0x61'
If you need to work with values larger than a byte, you could create the mask from the int.bit_length()
method on your value:
>>> value = 0x9E
>>> mask = (1 << value.bit_length()) - 1
>>> hex(value ^ mask)
'0x61'
>>> value = 0x9E9E
>>> mask = (1 << value.bit_length()) - 1
>>> hex(value ^ mask)
'0x6161'
Solution 2
Hah. just found out that python bin()
return a string!
so we can have some fun at this!
for x in numbers: # numbers is a list of int
b = bin(x)
#print b # e.g. String 0b1011100101
b = b.replace('0', 'x')
b = b.replace('1', '0')
b = b.replace('x', '1')
b = b.replace('1b', '0b')
#print b # String 0b0100011010
print int(b, 2) # the decimal representation
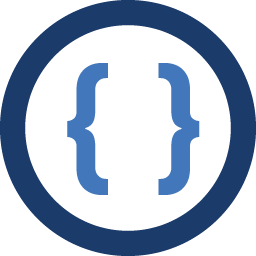
Author by
Admin
Updated on June 17, 2022Comments
-
Admin almost 2 years
Is there an easy way to produce a one's complement in python?
For instance, if you take the hex value
0x9E
, I need to convert it to0x61
.I need to swap the binary 1's for 0's and 0's for 1's. It feels like this should be simple.
-
Kos over 11 yearsOK I'm confused, why is
~x
wrong? It's supposed to be the binary negation operator after all. I can't wrap my mind over the lack of signed/unsigned distinction when binary ops are concerned -
Martijn Pieters over 11 years@Kos:
~
literally returns-(x+1)
, which is great when dealing with signed values. So~0x9E
is-159
, or-0x9f
hex. For unsigned bitwise work, not so great.. -
Kos over 11 yearsOK I got this, Python's "conceptually infinite string of
1
's in front of negative numbers" approach has messed with my head. Analogies to C bitwise ops won't work here :( -
Martijn Pieters over 11 years@aka.nice: Sure, that works (I used
|
bitwise or accidentally). You still need to create the mask though. -
Joe almost 4 yearsThis just made it to the top five of my most-pragmatic-code list :)