Future<Uint8List> to Base64 or PNG in Flutter
7,571
Solution 1
import 'dart:convert';
// async variant
final imageData = await _controller.toPngBytes(); // must be called in async method
final imageEncoded = base64.encode(imageData); // returns base64 string
// callback variant
_controller.toPngBytes().then((data) {
final imageEncoded = base64.encode(data);
});
Solution 2
This function made me upload an image to Xano correctly:
import 'dart:convert';
import 'dart:typed_data';
String uint8ListTob64(Uint8List uint8list) {
String base64String = base64Encode(uint8list);
String header = "data:image/png;base64,";
return header + base64String;
}
You can transform an image into Uint8List in the following way:
String path = "image.png";
File file = File(path);
Uint8List uint8list = file.readAsBytesSync();
//or
Uint8List uint8list = await file.readAsBytes();
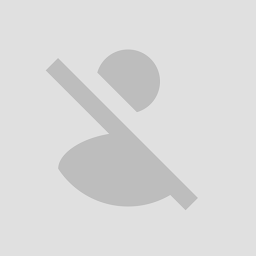
Author by
SerNet OE
Updated on December 26, 2022Comments
-
SerNet OE over 1 year
I need to convert a
Future<Uint8List>
to Base64 or PNG in Flutter, I am using this pub to get signature and export it but when I calltoPngBytes()
method (method in pub) it returns aFuture<Uint8List>
and I need to convert it toBase64
format orList<int>
at leastByteData
format, I can not convert it to a more usable format for me, can anyone help me to resolve this._controller.toPngBytes(); // _controller is a variable that holds info about my signature.
-
pskink over 3 yearswhat is wrong with
Uint8List
? -
SerNet OE over 3 yearsBasicly not usefull in my case
-
pskink over 3 yearsand
ByteData
is more useful? if so, use ByteData.sublistView constructor -
SerNet OE over 3 yearsActually
List<int>
is a lot more useful for me to usebase64Encode()
, I am not familiar with ` ByteData.sublistView` can you give an example for my case please? -
pskink over 3 years"Actually List<int> is a lot more useful for me " - Uint8List is a
List<int>
- seeImplemented types
it the link i posted
-
-
SerNet OE over 3 yearsOf courese I tried it but it is not appliciable because
toPngBytes()
returnsFuture<Uint8List>
butbase64Encode()
takes paremeter typeList<int>
-
BambinoUA over 3 yearsDid you read the comments? Do you know what is async/await? Update answer.
-
SerNet OE over 3 yearsYes, I read them but I did not understand why I should call it in async method but now, I understand it thanks for helping, now I know how to use Future variables.
-
saddam about 2 yearshow to convert Uint8List to Image dataType.