Generate random numbers following a normal distribution in C/C++
Solution 1
There are many methods to generate Gaussian-distributed numbers from a regular RNG.
The Box-Muller transform is commonly used. It correctly produces values with a normal distribution. The math is easy. You generate two (uniform) random numbers, and by applying an formula to them, you get two normally distributed random numbers. Return one, and save the other for the next request for a random number.
Solution 2
C++11
C++11 offers std::normal_distribution
, which is the way I would go today.
C or older C++
Here are some solutions in order of ascending complexity:
Add 12 uniform random numbers from 0 to 1 and subtract 6. This will match mean and standard deviation of a normal variable. An obvious drawback is that the range is limited to ±6 – unlike a true normal distribution.
The Box-Muller transform. This is listed above, and is relatively simple to implement. If you need very precise samples, however, be aware that the Box-Muller transform combined with some uniform generators suffers from an anomaly called Neave Effect1.
For best precision, I suggest drawing uniforms and applying the inverse cumulative normal distribution to arrive at normally distributed variates. Here is a very good algorithm for inverse cumulative normal distributions.
1. H. R. Neave, “On using the Box-Muller transformation with multiplicative congruential pseudorandom number generators,” Applied Statistics, 22, 92-97, 1973
Solution 3
A quick and easy method is just to sum a number of evenly distributed random numbers and take their average. See the Central Limit Theorem for a full explanation of why this works.
Solution 4
I created a C++ open source project for normally distributed random number generation benchmark.
It compares several algorithms, including
- Central limit theorem method
- Box-Muller transform
- Marsaglia polar method
- Ziggurat algorithm
- Inverse transform sampling method.
-
cpp11random
uses C++11std::normal_distribution
withstd::minstd_rand
(it is actually Box-Muller transform in clang).
The results of single-precision (float
) version on iMac [email protected] , clang 6.1, 64-bit:
For correctness, the program verifies the mean, standard deviation, skewness and kurtosis of the samples. It was found that CLT method by summing 4, 8 or 16 uniform numbers do not have good kurtosis as the other methods.
Ziggurat algorithm has better performance than the others. However, it does not suitable for SIMD parallelism as it needs table lookup and branches. Box-Muller with SSE2/AVX instruction set is much faster (x1.79, x2.99) than non-SIMD version of ziggurat algorithm.
Therefore, I will suggest using Box-Muller for architecture with SIMD instruction sets, and may be ziggurat otherwise.
P.S. the benchmark uses a simplest LCG PRNG for generating uniform distributed random numbers. So it may not be sufficient for some applications. But the performance comparison should be fair because all implementations uses the same PRNG, so the benchmark mainly tests the performance of the transformation.
Solution 5
Here's a C++ example, based on some of the references. This is quick and dirty, you are better off not re-inventing and using the boost library.
#include "math.h" // for RAND, and rand
double sampleNormal() {
double u = ((double) rand() / (RAND_MAX)) * 2 - 1;
double v = ((double) rand() / (RAND_MAX)) * 2 - 1;
double r = u * u + v * v;
if (r == 0 || r > 1) return sampleNormal();
double c = sqrt(-2 * log(r) / r);
return u * c;
}
You can use a Q-Q plot to examine the results and see how well it approximates a real normal distribution (rank your samples 1..x, turn the ranks into proportions of total count of x ie. how many samples, get the z-values and plot them. An upwards straight line is the desired result).
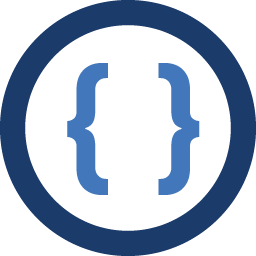
Admin
Updated on January 07, 2021Comments
-
Admin over 3 years
How can I easily generate random numbers following a normal distribution in C or C++?
I don't want any use of Boost.
I know that Knuth talks about this at length but I don't have his books at hand right now.
-
JoeG about 14 yearsHe didn't ask for standard, he asked for 'not boost'.
-
Rich about 14 yearsIf you need speed, then the polar method is faster, though. And the Ziggurat algorithm even more (albeit much more complex to write).
-
Morlock about 14 years+1 Very interesting approach. Is it verified to really give normally distributed sub ensembles for smaller groups?
-
Paul R about 14 years@Morlock The larger the number of samples you average the closer you get to a Gaussian distribution. If your application has strict requirements for the accuracy of the distribution then you might be better off using something more rigorous, like Box-Muller, but for many applications, e.g. generating white noise for audio applications, you can get away with a fairly small number of averaged samples (e.g. 16).
-
Morlock about 14 yearsPlus, how do you parametrize this to get a certain amount of variance, say you want a mean of 10 with a standard deviation of 1?
-
user1066101 about 14 years@Morlock: To get a given mean and SD you scale your underlying samples properly. Generally, the underlying samples, u, are uniform over 0 to 1. Make them uniform over -1 to +1 (compute 2 u -1). You can then add the desired mean and multiply to get the desired standard deviation.
-
Paul R about 14 years@Morlock If you scale your PRNG to give random numbers in the range -1.0 to +1.0 then you get a mean of 0.0 and a variance of 0.5 when you take a sufficiently large average. You can just linearly shift and scale either the input numbers or the output average to get whatever mean and variance you need.
-
Morlock about 14 years@S.Lott I can easily see how to scale a distribution given a known SD and mean, as you suggest, but it looks a lot less straight forward to do so with a distribution generated using the central limit theorem, given that 3 values will affect the level of stochasticity: the number of 1) original random numbers; 2) values used in the averages; 3) averages done. I guess you must do quite a number of trials (1000s) to parametrize the whole thing before you can be comfortable to scale it appropriately :) Not that it would necessarily be very heavy on computation time. Cheers!
-
pyCthon over 12 yearsby any chance would you have another link to the pdf on the Neave effect? or the original journal article reference? thank you
-
Peter G. over 12 years@stonybrooknick The original reference is added. Cool remark: While googling "box muller neave" to find the reference, this very stackoverflow question came up on the first result page!
-
pyCthon over 12 yearsyeah its not every well known outside certain small communities and interest groups
-
solvingPuzzles almost 12 yearsWhat is sampleNormalManual()?
-
Pete855217 almost 12 years@solvingPuzzles - sorry, corrected the code. It's a recursive call.
-
Petter over 11 yearsThis is a very inefficient method of generating samples from a normal distribution. I would definitely not call it "quick."
-
Paul R over 11 years@Ben: it depends on how many averages you need to take - if you only need approximately normal distribution and can use say N = 16 averaged numbers then it is much more efficient, if less accurate, than methods that use transcendental functions,
-
Petter over 11 years@Paul: I would still not call this "quick." "Easy," yes. There are correct algorithms that would beat this even for N = 16.
-
Paul R over 11 years@Ben: could you point me at an efficient algo for this ? I've only ever used the averaging technique for generating approximately Gaussian noise for audio and image processing with real-time constraints - if there's a way of achieving this in fewer clock cycles then that could be very useful.
-
Mat about 11 yearsThat's already been mentioned by Ben (stackoverflow.com/a/11977979/635608)
-
dwbrito almost 11 yearsfound an implementation of the Ziggurat here people.sc.fsu.edu/~jburkardt/c_src/ziggurat/ziggurat.html It's quite complete.
-
Admin over 10 yearsNote, C++11 adds
std::normal_distribution
which does exactly what you ask for without delving into mathematical details. -
Petter over 10 years-1 Not working for e.g. RANDN2(0.0, d + 1.0). Macros are notorious for this.
-
the swine over 10 yearsThis is bound to crash at some rare event (showcasing application to your boss rings a bell?). This should be implemented using a loop, not using recursion. The method looks unfamiliar. What is the source / how is it called?
-
Pete855217 over 10 yearsBox-Muller transcribed from a java implementation. As I said, it's quick and dirty, feel free to fix it.
-
Pete855217 over 10 years@Peter G. Why would anyone downvote your answer? - possibly the same person did my comment below too, which I'm fine with, but I thought your answer was very good. It would be good if SO made downvotes force a real comment..I suspect most downvotes of old topics are just frivolous and trolly.
-
interDist over 10 yearsThe macro will fail if
rand()
ofRANDU
returns a zero, since Ln(0) is undefined. -
HelloGoodbye about 10 yearsHave you actually tried this code? It looks like you have created a function that generates numbers which are Rayleigh distributed. Compare to the Box–Muller transform, where they multiply with
cos(2*pi*rand/RAND_MAX)
, whereas you multiply with(rand()%2 ? -1.0 : 1.0)
. -
Petter over 9 years@PaulR: The answers below (std::normal_distribution) are generally much better.
-
Paul R over 9 years@Petter: you're probably right in the general case, for floating point values. There are still application areas like audio though, where you want fast integer (or fixed point) gaussian noise, and accuracy is not too important, where the simple averaging method is more efficient and useful (especially for embedded applications, where there may not even be hardware floating point support).
-
Walter over 8 yearsthe
generator
should really be seeded. -
Petter over 8 yearsIt is always seeded. There is a default seed.
-
Octopus over 8 yearsSeems perfectly reasonable and gives good results. I implemented this in javascript (and tested the distribution).
-
Pete855217 over 8 yearsI found it worked well too Octopus, validating its results with a Q-Q plot. Just be aware it involves a recursive call, the number of calls being subject to rand(), so theoretically it runs for an 'unknown' number of iterations, though I've never had any problems. A better solution is of course to use a library like boost, but if you must have a home-grown, or quick method, it works fine.
-
Konstantin Burlachenko over 8 years"Add 12 uniform numbers from 0-1 and subtract 6." -- distribution of this variable will have normal distribution? Can you provide a link with derivation, because during derivation central limit theorem, n->+inf is very need assumption.
-
Peter G. over 8 years@bruzias, adding 12 uniform numbers from 0 to 1 and subtracting isn't normally distributed, it only results in the same mean (i.e. 0) and standard deviation (i.e. sqrt( 12*1/12) = 1, because the SD of uniform distribution from 0 to 1 is 1/12 and variances of independent variables add).
-
Arno Duvenhage over 8 yearsstd::normal_distribution is not guaranteed to be consistent across all platforms. I'm doing the tests now, and MSVC provides a different set of values from, for example, Clang. The C++11 engines seem to generate the same sequences (given the same seed), but the C++11 distributions seem to be implemented using different algorithms on different platforms.
-
Konstantin Burlachenko over 6 yearsI have several downvotes. Let me know what is bad here?
-
greggo over 6 years"But the performance comparison should be fair because all implementations uses the same PRNG" .. Except that BM uses one input RN per output, whereas CLT uses many more, etc... so the time to generate a uniform random # matters.
-
greggo over 6 yearsFWIW, many compilers will be able to turn that particular recursive call into a 'jump to top of function'. Question is whether you want to count on it :-) Also, probability that it takes > 10 iterations is 1 in 4.8 million. p(>20) is the square of that, etc.
-
user2820579 about 6 yearsThe question is how to generate pseudo random numbers in the computer (I know, language is loose here), it is not a question of mathematical existence.
-
Konstantin Burlachenko about 6 yearsYes, you're right. And the answer is how to generate pseudo random number with normal distribution based on generator which has uniform distribution. Source code have been provided, you can rewrite it in any language.
-
user2820579 about 6 yearsSure, I think the guy es looking for e.g. "Numerical Recipes in C/C++". By the way, just to complement our discussion, the authors of this last book give interesting references for a couple of pseudo-random generators that fulfills standards for being "decent" generators.
-
Denise Skidmore about 6 yearsWould adding more points to the sample decrease standard deviation? You're more likely to pull towards median with more samples. True bell curves don't have upper and lower limits like the random number generator will do, so there are some discrepancies at the very ends of the curve here.
-
Paul R about 6 years@DeniseSkidmore: yes, see comments above - this method is far from perfect but it’s still useful for certain cases where the approximation is good enough, e.g. generating noise for audio applications.
-
Konstantin Burlachenko about 6 yearsIt's time to save this post before it have been deleted from StackOverflow
-
Konstantin Burlachenko about 6 yearsI made backup here: sites.google.com/site/burlachenkok/download
-
Konstantin Burlachenko over 5 years@Peter what do you think about method that I descrived above (with "-3") score above?
-
Peter G. over 5 yearsThe method you described in 2016 is as far as I see it the Box-Muller Transform. The Box-Muller Transform was given as an answer already in 2010. So, I guess people downvoted because 6 years later, you answered a question which already had been answered with your solution.
-
angstyloop almost 2 yearspeople.sc.fsu.edu/~jburkardt/c_src/normal/normal.c thanks @dwbrito