Generating a number between 1 and 2 java Math.random()
Solution 1
If you want the values 1 or 2 with equal probability, then int temp = (Math.random() <= 0.5) ? 1 : 2;
is all you need. That gives you a 1 or a 2, each with probability 1/2.
Solution 2
int tmp = (int) ( Math.random() * 2 + 1); // will return either 1 or 2
Math.random()
returns numbers from [0, 1)
. Because (int)
always rounds down (floors), you actually want the range [1,3)
, so that [1,2)
will be rounded to 1 and [2,3)
will be rounded to 2.
Solution 3
Try this.
int tmp = (int)( Math.floor( Math.random() + 1.5 ) )
Math.random() -> [0, 1)
Math.random() + 1.5 -> [1.5, 2.5)
So when you take the floor, it is either 1 or 2 with equal
probability because [1.5, 2.5) = [1.5, 2) U [2, 2.5)
and length([1.5, 2)) = length([2, 2.5)) = 0.5
.
Solution 4
Ok, you want to use Math.random
, but you could use java.util.Random
so no casting or rounding is required:
java.util.Random random = new java.util.Random();
int tmp = random.nextInt(2) + 1;
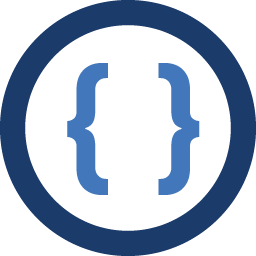
Admin
Updated on January 21, 2020Comments
-
Admin over 4 years
I'm working on creating a range of numbers between 1 and 2 using the imported math.random() class.
Here's how I got it working so far, however I can't see how it would work:
int tmp = (int)(Math.random()*1)+1;
Anyonould?e know if that actually works to get the range? if not.. then what
EDIT: Looking for either the number 1 or 2.
-
peter.petrov over 10 yearsNot really, you should do < 0.5 and not <= 0.5 for equal probability, right?
-
pjs over 10 years@peter.petrov Mathematically it's discrete inversion of the CDF, and CDF's are
<=
inequalities. Computationally you could argue that the RNG yields 0 but not 1, so there's an asymmetry to the tune of one part in 2**31-1, which practically speaking makes no difference. Since I'm a statistician by trade, I tend to go with a straight-up translation the mathematical definition. -
Adam Gent over 10 years+1 I was looking at these answers and was wondering why no one was using
Random
. Especially since you can replace it withSecureRandom
. -
Admin over 10 yearsI'm using this code for a class, we're supposed to say in the curriculum and math.random() was the only random taught.
-
pjs over 10 years@peter.petrov This also would lend itself easily to splitting the mix to a different proportion. Simply change the proportion value in the test to a new target.
-
Raul Guiu over 10 yearsIt is part of
java.util
, that class is there to solve this kind of problems. -
pjs over 10 years@peter.petrov I think you could argue that mathematically you have two equivalent ranges, while technically I don't. Yours requires more computation by a hair (addition, floor, and casting vs a single comparison). I don't think any statistical test could tell the difference in the results based on any sample size people are actually likely to draw.
-
peter.petrov over 10 yearsOK, I think I see. Thanks.
-
Dawood ibn Kareem over 10 years+1, but you don't need the
Math.floor
. -
ajb over 10 years@pjs I think the asymmetry is more like one part in 252-1, not 231-1.
Math.random
returns adouble
which has 52 significant bits of precision. -
pjs over 10 years@ajb Thanks for the info. I think the bottom line is that nobody would be able to tell the difference whether you use a
<
or a<=
on this one.