Get absolute path with boost::filesystem::path
Solution 1
You say you want an absolute path, but your example shows that you already have an absolute path. The process of removing the ..
components of a path is known as canonicalization. For that, you should call canonical
. It happens to also perform the task of absolute
, so you don't need to call absolute
or make_absolute
first. The make_absolute
function requires a base path; you can pass it current_path()
if you don't have anything better.
Solution 2
Update, since this still appears to be Google's top hit concerning absolute paths:
As of Boost 1.57, some of the previously suggested functions have since been removed.
The solution that worked for me was
boost::filesystem::path canonicalPath = boost::filesystem::canonical(previousPath, relativeTo);
(using the free-standing method canonical(), defined in boost/filesystem/operations.hpp, which is automatically included via boost/filesystem.hpp)
Important: calling canonical on a path that does not exist (e.g., you want to create a file) will throw an exception. In that case, your next best bet is probably boost::filesystem::absolute(). It will also work for non-existing paths, but won't get rid of dots in the middle of the path (as in a/b/c/../../d.txt). Note: Make sure relativeTo refers to a directory, calling parent_path() on paths referring to files (e.g. the opened file that contained a directory or file path relative to itself).
Solution 3
The documentation shows that the make_absolute
has an optional second parameter that defaults to your current path:
path absolute(const path& p, const path& base=current_path());
Try it without the second parameter and see if it returns the results you're looking for.
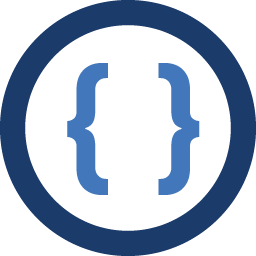
Admin
Updated on January 15, 2021Comments
-
Admin over 3 years
My current working directory is located at
/home/myuser/program
, I created aboost::filesystem::path
object pointing to it. I appended/../somedir
so it becomes/home/myuser/program/../somedir
. But I need to get its resolved absolute path, which would be/home/myuser/somedir
.I have been trying for long time and I do not find any method in their reference to do this. There is a method called
make_absolute
, which seems to be supposed to do what I expect, but I have to give it a “root” path argument. Which should it be? do I really need to do this to get the real absolute path? Is there any other way?