Get class of generic
Solution 1
You can provide the type dynamically, however the compiler doesn't do this for you automagically.
public abstract class LastActionHero<H extends Hero>(){
protected final Class<H> hClass;
protected LastActionHero(Class<H> hClass) {
this.hClass = hClass;
}
// use hClass how you like.
}
BTW: It not impossible to get this dynamically, but it depends on how it is used. e.g
public class Arnie extends LastActionHero<MuscleHero> { }
It is possible to determine that Arnie.class has a super class with a Generic parameter of MuscleHero.
public class Arnie<H extend Hero> extends LastActionHero<H> { }
The generic parameter of the super class will be just H
in this case.
Solution 2
We do it in the following way:
private Class<T> persistentClass;
public Class<T> getPersistentClass() {
if (persistentClass == null) {
this.persistentClass = (Class<T>) ((ParameterizedType) getClass().getGenericSuperclass()).getActualTypeArguments()[0];
}
return persistentClass;
}
Solution 3
One way is to keep reference to your parameterized type like having an attribute of
private Class<H> clazz;
And create a setter or a constructor that takes in a Class<H>
.
Parameterized Types are erased at runtime, hence why you can't do what you ask.
Solution 4
You can do it without passing in the class:
public abstract class LastActionHero<H extends Hero>() {
Class<H> clazz = (Class<H>) DAOUtil.getTypeArguments(LastActionHero.class, this.getClass()).get(0);
}
You need two functions from this file: http://code.google.com/p/hibernate-generic-dao/source/browse/trunk/dao/src/main/java/com/googlecode/genericdao/dao/DAOUtil.java
For more explanation: http://www.artima.com/weblogs/viewpost.jsp?thread=208860
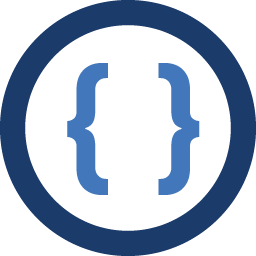
Admin
Updated on August 04, 2022Comments
-
Admin almost 2 years
My class starts with
public abstract class LastActionHero<H extends Hero>(){
Now somewhere in the code I want to write
H.class
but that isn't possible (likeString.class
orInteger.class
is).Can you tell me how I can get the
Class
of the generic?