Get data from pandas into a SQL server with PYODBC
Solution 1
For the 'write to sql server' part, you can use the convenient to_sql
method of pandas (so no need to iterate over the rows and do the insert manually). See the docs on interacting with SQL databases with pandas: http://pandas.pydata.org/pandas-docs/stable/io.html#io-sql
You will need at least pandas 0.14 to have this working, and you also need sqlalchemy
installed. An example, assuming df
is the DataFrame you got from read_table
:
import sqlalchemy
import pyodbc
engine = sqlalchemy.create_engine("mssql+pyodbc://<username>:<password>@<dsnname>")
# write the DataFrame to a table in the sql database
df.to_sql("table_name", engine)
See also the documentation page of to_sql
.
More info on how to create the connection engine with sqlalchemy for sql server with pyobdc, you can find here:http://docs.sqlalchemy.org/en/rel_1_1/dialects/mssql.html#dialect-mssql-pyodbc-connect
But if your goal is to just get the csv data into the SQL database, you could also consider doing this directly from SQL. See eg Import CSV file into SQL Server
Solution 2
Python3 version using a LocalDB SQL instance:
from sqlalchemy import create_engine
import urllib
import pyodbc
import pandas as pd
df = pd.read_csv("./data.csv")
quoted = urllib.parse.quote_plus("DRIVER={SQL Server Native Client 11.0};SERVER=(localDb)\ProjectsV14;DATABASE=database")
engine = create_engine('mssql+pyodbc:///?odbc_connect={}'.format(quoted))
df.to_sql('TargetTable', schema='dbo', con = engine)
result = engine.execute('SELECT COUNT(*) FROM [dbo].[TargetTable]')
result.fetchall()
Solution 3
Yes, the bcp
utility seems to be the best solution for most cases.
If you want to stay within Python, the following code should work.
from sqlalchemy import create_engine
import urllib
import pyodbc
quoted = urllib.parse.quote_plus("DRIVER={SQL Server};SERVER=YOUR\ServerName;DATABASE=YOur_Database")
engine = create_engine('mssql+pyodbc:///?odbc_connect={}'.format(quoted))
df.to_sql('Table_Name', schema='dbo', con = engine, chunksize=200, method='multi', index=False, if_exists='replace')
Don't avoid method='multi'
, because it significantly reduces the task execution time.
Sometimes you may encounter the following error.
ProgrammingError: ('42000', '[42000] [Microsoft][ODBC SQL Server Driver][SQL Server]The incoming request has too many parameters. The server supports a maximum of 2100 parameters. Reduce the number of parameters and resend the request. (8003) (SQLExecDirectW)')
In such a case, determine the number of columns in your dataframe: df.shape[1]
. Divide the maximum supported number of parameters by this value and use the result's floor as a chunk size.
Solution 4
I found that using bcp utility (https://docs.microsoft.com/en-us/sql/tools/bcp-utility) works best when you have a large dataset. I have 2.7 million rows that inserts at 80K rows/sec. You can store your data frame as csv file (use tabs for separator if your data doesn't have tabs and utf8 encoding). With bcp, I've used format "-c" and it works without issues so far.
Solution 5
This worked for me on Python 3.5.2:
import sqlalchemy as sa
import urllib
import pyodbc
conn= urllib.parse.quote_plus('DRIVER={ODBC Driver 17 for SQL Server};SERVER='+server+';DATABASE='+database+';UID='+username+';PWD='+ password)
engine = sa.create_engine('mssql+pyodbc:///?odbc_connect={}'.format(conn))
frame.to_sql("myTable", engine, schema='dbo', if_exists='append', index=False, index_label='myField')
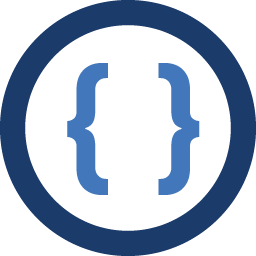
Admin
Updated on January 22, 2022Comments
-
Admin over 2 years
I am trying to understand how python could pull data from an FTP server into pandas then move this into SQL server. My code here is very rudimentary to say the least and I am looking for any advice or help at all. I have tried to load the data from the FTP server first which works fine.... If I then remove this code and change it to a select from ms sql server it is fine so the connection string works, but the insertion into the SQL server seems to be causing problems.
import pyodbc import pandas from ftplib import FTP from StringIO import StringIO import csv ftp = FTP ('ftp.xyz.com','user','pass' ) ftp.set_pasv(True) r = StringIO() ftp.retrbinary('filname.csv', r.write) pandas.read_table (r.getvalue(), delimiter=',') connStr = ('DRIVER={SQL Server Native Client 10.0};SERVER=localhost;DATABASE=TESTFEED;UID=sa;PWD=pass') conn = pyodbc.connect(connStr) cursor = conn.cursor() cursor.execute("INSERT INTO dbo.tblImport(Startdt, Enddt, x,y,z,)" "VALUES (x,x,x,x,x,x,x,x,x,x.x,x)") cursor.close() conn.commit() conn.close() print"Script has successfully run!"
When I remove the ftp code this runs perfectly, but I do not understand how to make the next jump to get this into Microsoft SQL server, or even if it is possible without saving into a file first.
-
Admin over 9 yearsthanks for the info apologies on late reply I will work with this and I am sure it will be ok.
-
Richard Blackman over 9 yearsi had to pass in engine.raw_connection() as explained here stackoverflow.com/questions/20401392/…
-
joris over 9 years@RichardBlackman That is not correct for pandas versions 0.14 and above. In that case, you should just pass the engine itself (but, the answer you linked to is relevant for pandas <= 0.13)
-
Some Guy about 7 years@joris , please update the answer, the doc page linked suggests starting 1.0.0 you need to explicity specify a driver,
create_engine("mssql+pyodbc://scott:tiger@myhost:port/databasename?driver=SQL+Server+Native+Client+10.0")
I was trying to connect with the engine without specifying one and my to_sql kept complaining that I didn't choose a driver -
joris about 7 years@SomeGuy Thanks for noting, I updated the link to the sqlalchemy docs to link to a newer version.
-
Babu Arunachalam over 5 yearsbcp tends to throw errors with types and formatting in the csv file. Using a separator like "|" (remove it from data) works better than ",". It is better to have the target table as all varchar columns so that bcp doesn't throw errors. You can then run an "insert into" to your target table with the correct types by casting it.
-
Serhii Kushchenko over 4 yearsThis way of connecting Python to MS SQL Server was the best in my case
-
Nabakamal Das over 3 yearsI had to add the "Trusted Connection" value. "DRIVER={SQL Server Native Client 11.0};SERVER=.;DATABASE=MyDB;Trusted_Connection=yes;"
-
Admin over 2 yearsAs it’s currently written, your answer is unclear. Please edit to add additional details that will help others understand how this addresses the question asked. You can find more information on how to write good answers in the help center.