Get file size in KB/MB/GB in windows batch file
Just as an alternative, you can use the output of robocopy
command to retrieve the required information
@echo off
setlocal enableextensions disabledelayedexpansion
set "file=d:\somewhere\file.ext"
for %%z in ("%file%") do for /f "tokens=1,2" %%a in ('
robocopy "%%~dpz." "%%~dpz." "%%~nxz" /l /nocopy /is /njh /njs /ndl /nc
') do if "%%~dz"=="%%~db" (
echo "%%~z" : [%%a]
) else (
echo "%%~z" : [%%a%%b]
)
robocopy
will be used to only list (/l
) the information of the file being copied, removing any non needed information from the output (the rest of the switches) and using a for /f
command to tokenize the output lines and read only the file size
In the case of folders, the same idea can be used, but instead of reading the data from the file list, we can use a recursive "copy" and retrieve the data from the job summary
@echo off
setlocal enableextensions disabledelayedexpansion
set "folder=%~f1" & if not defined folder set "folder=%cd%"
set "size=" & for %%z in ("%folder%") do for /f "skip=2 tokens=2,3 delims=: " %%a in ('
robocopy "%%~fz\." "%%~fz\." /l /nocopy /s /is /njh /nfl /ndl /r:0 /w:0 /xjd /xjf /np
^| find ":"
') do if not defined size (
(for /f "delims=0123456789." %%c in ("%%b") do (break)) && (
set "size=%%a%%b"
) || (
set "size=%%a"
)
)
echo "%folder%" : [%size%]
Switches changed to retrive only the job summary, and filtered to only read the line containing the Bytes
information.
edited 2016/10/26 - changed robocopy switches to face permission problems, changed Byte:
retrieval to :
line filter plus skip clause just in case there is a locale without the Bytes:
string, changed storage unit detection.
Related videos on Youtube
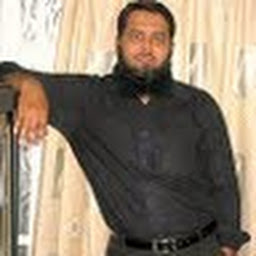
Syed Jahanzaib
Updated on September 18, 2022Comments
-
Syed Jahanzaib over 1 year
I have a Windows (Windows 7/2008 R2) batch file which takes daily backup of file.(Oracle dump export file e.g dump-2016-10-17.DMP)
I want to add the FILE size (in a variable) which should be in KB/MB/GB according to the size, or at least it should get file size in MB. CODE:
@echo off SET DONE=0 SET Bytes=%~z1 SET KB=%Bytes:~0,-3% SET MB=%Bytes:~0,-6% SET GB=%Bytes:~0,-9% SET TB=%Bytes:~0,-12% rem echo %~f1 IF "%KB%" EQU "" SET DONE=B IF %DONE% EQU B echo Size is... %Bytes% Bytes IF %DONE% EQU B GOTO END IF "%MB%" EQU "" SET DONE=K IF %DONE% EQU K SET /a KB=(%BYTES%/1024)+1 IF %DONE% EQU K echo Size is... %KB% KB IF %DONE% EQU K GOTO END IF "%GB%" EQU "" SET DONE=M IF %DONE% EQU M SET /a MB=(%BYTES%/1048576)+1 IF %DONE% EQU M echo Size is... %MB% MB IF %DONE% EQU M GOTO END IF "%TB%" EQU "" SET DONE=M IF %DONE% EQU M SET /a MB=%KB%/1049 IF %DONE% EQU M echo Size is... %MB% MB (approx) IF %DONE% EQU M GOTO END SET DONE=G IF %DONE% EQU G SET /a GB=%MB%/1074 IF %DONE% EQU G echo Size is... %GB% GB (approx) IF %DONE% EQU G GOTO END :END
-
Syed Jahanzaib over 7 yearsHow i can use the same batch command to get folder size?
-
MC ND over 7 years@SyedJahanzaib, What do you mean with "folder size"? Files inside the folder? , files under the folder and any subfolder?, ...?
-
Syed Jahanzaib over 7 yearsexample i want to get full size of D:\temp folder. There could be sub directories in it like D:\temp\1 d:\temp\2 So I want full size of the D:\temp folder.
-
Syed Jahanzaib over 7 yearsresult should be single entry like D:\temp = size = 900M or 5GB
-
MC ND over 7 years@SyedJahanzaib, answer updated.
-
Syed Jahanzaib over 7 yearsthe code to list overall folder size (including subfolders too) is not displaying any size, in fact the code is not returning any output. just blank
-
MC ND over 7 years@SyedJahanzaib, Tested on two different systems with two different locales and working. Anyway I've changed the code to face posible problems with filesystem access restrictions or locale conflicts.
-
Syed Jahanzaib over 7 yearsI have tried the updated code in windows 2008 and win 7 / 64bit. with admin account. I tried updated code and its returning following result. D:\zaib>size.bat "D:\zaib" : [:] I tried to add folder as well like size.bat d:\folder1 but still same. with previous code, it was not returning any kind of result just blank.
-
MC ND over 7 years@SyedJahanzaib, from your output, in your locale the output of the
robocopy
command has space(s) between theBytes
string and the colon, something that is not present in the locales I have access to. I've changed thefor /f %%a
to include adelims
clause to use include the colons as delimiters instead of handling them as tokens. -
Syed Jahanzaib over 7 yearsThis last updated Worked Perfectly. Many thanks for your time and efforts. Now i can use this in my batch file of backup that will include dir size as well. Thx :) Output: D:\zaib>size.bat "D:\zaib" : [1.728g]