Get frame to Hikvision IP Camera in python
Solution 1
Go to your camera settings (network configuration) and check de rtsp port, in my case is 555.
I have a hikvision DS-2CD2D14WD, in my case I had to use the following:
rtsp://yourusername:[email protected]:555/Streaming/channels/2/
You can test your configuration in a program like VLC player.
The path after the IP address can change depending of your model, check
this page for other hikvision models:
https://www.ispyconnect.com/man.aspx?n=Hikvision .
I recomend you to check this github page:
https://github.com/JFF-Bohdan/pynvr.git
Please, Let me know if this information works for you.
Example:
import numpy as np
import cv2
cap = cv2.VideoCapture()
cap.open("rtsp://yourusername:[email protected]:555/Streaming/channels/2/")
while(True):
# Capture frame-by-frame
ret, frame = cap.read()
# Our operations on the frame come here
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# Display the resulting frame
cv2.imshow('frame',ret)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# When everything done, release the capture
cap.release()
cv2.destroyAllWindows()
Solution 2
I get this on hikvisionapi-PyPI
from hikvisionapi import Client
import cv2
import time
cam = Client('http://192.168.1.10', 'admin', 'Astra123', timeout=30)
cam.count_events = 2 # The number of events we want to retrieve (default = 1)
response = cam.Streaming.channels[102].picture(method='get', type='opaque_data')
with open('screen.jpg', 'wb') as f:
for chunk in response.iter_content(chunk_size=1024):
if chunk:
f.write(chunk)
time.sleep(1)
img = cv2.imread('screen.jpg')
cv2.imshow("show", img)
cv2.waitKey(0)
Solution 3
This should be the one you are looking for.
import cv2
import requests
import numpy as np
from hikvisionapi import Client
cam = Client('http://192.168.1.10', 'admin', 'Astra123')
while True:
print("starting picture capture")
vid = cam.Streaming.channels[102].picture(method ='get', type = 'opaque_data')
bytes = b''
with open('screen.jpg', 'wb') as f:
for chunk in vid.iter_content(chunk_size=1024):
bytes += chunk
a = bytes.find(b'\xff\xd8')
b = bytes.find(b'\xff\xd9')
if a != -1 and b != -1:
jpg = bytes[a:b+2]
bytes = bytes[b+2:]
i = cv2.imdecode(np.fromstring(jpg, dtype=np.uint8), cv2.IMREAD_COLOR)
cv2.imshow('i', i)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
if cv2.waitKey(0) == 27:
cv2.destroyAllWindows()
break
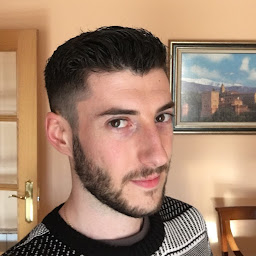
Francisco Pérez
Updated on June 26, 2022Comments
-
Francisco Pérez almost 2 years
I have the next Hikvision IP Camera http://www.hikvision.com/es/Products_accessries_161_i11969.html I configured it in my local network and I have connection with the browser and I can see the camera working perfectly. I have searched in different post to get frame in cameras like this, but I can't do it. Here I put the code that I used to try it and the response. I want to obtain a frame and save it in my destock to processed after.
import cv2 import requests import numpy as np import urllib import hikvision.api import requests import shutil #Donwload from: https://github.com/fbradyirl/hikvision/archive/master.zip hik_camera = hikvision.api.CreateDevice('192.168.1.64', username='admin', password=contrasenia) """ INFO:hikvision.api:Initialising new hikvision camera client INFO:hikvision.api:motion_url: http://192.168.1.64/MotionDetection/1 INFO:hikvision.api:ElementTree.register_namespace: http://www.hikvision.com/ver10/XMLSchema INFO:hikvision.api:Going to probe device to test connection INFO:hikvision.api:url: http://192.168.1.64/System/deviceInfo INFO:requests.packages.urllib3.connectionpool:Starting new HTTP connection (1): 192.168.1.64 DEBUG:requests.packages.urllib3.connectionpool:"GET /System/deviceInfo HTTP/1.1" 200 696 DEBUG:hikvision.api:response: <Response [200]> DEBUG:hikvision.api:status_code 200 DEBUG:hikvision.api:element_to_query: .//{http://www.hikvision.com/ver10/XMLSchema}firmwareVersion result: <Element '{http://www.hikvision.com/ver10/XMLSchema}firmwareVersion' at 0x7fb090b76cc8> INFO:requests.packages.urllib3.connectionpool:Starting new HTTP connection (1): 192.168.1.64 DEBUG:requests.packages.urllib3.connectionpool:"GET /MotionDetection/1 HTTP/1.1" 200 407 DEBUG:hikvision.api:Response: <?xml version="1.0" encoding="UTF-8"?> <MotionDetection version="1.0" xmlns="http://www.hikvision.com/ver10/XMLSchema"> <id>1</id> <enabled>false</enabled> <regionType>grid</regionType> <Grid> <rowGranularity>18</rowGranularity> <columnGranularity>22</columnGranularity> </Grid> <MotionDetectionRegionList> <sensitivityLevel>0</sensitivityLevel> </MotionDetectionRegionList> </MotionDetection> INFO:hikvision.api:Current motion detection state? enabled: false WARNING:hikvision.api:sensitivityLevel is 0. INFO:hikvision.api:sensitivityLevel now set to 1 INFO:hikvision.api:Connected OK! INFO:hikvision.api:Camera firmaward version: V5.4.3 INFO:hikvision.api:Motion Detection enabled: False """ #The method: """ hik_camera.__class__( hik_camera.__setattr__( hik_camera.__delattr__( hik_camera.__sizeof__( hik_camera.__dict__ hik_camera.__str__( hik_camera.__dir__( hik_camera.__subclasshook__( hik_camera.__doc__ hik_camera.__weakref__ hik_camera.__eq__( hik_camera._base hik_camera.__format__( hik_camera._host hik_camera.__ge__( hik_camera._password hik_camera.__getattribute__( hik_camera._sensitivity_level hik_camera.__gt__( hik_camera._username hik_camera.__hash__( hik_camera._xml_namespace hik_camera.__init__( hik_camera.disable_motion_detection( hik_camera.__le__( hik_camera.enable_motion_detection( hik_camera.__lt__( hik_camera.get_about( hik_camera.__module__ hik_camera.get_version( hik_camera.__ne__( hik_camera.is_motion_detection_enabled( hik_camera.__new__( hik_camera.motion_url hik_camera.__reduce__( hik_camera.put_motion_detection_xml( hik_camera.__reduce_ex__( hik_camera.xml_motion_detection_off hik_camera.__repr__( hik_camera.xml_motion_detection_on """ hik_camera #<hikvision.api.CreateDevice object at 0x7fb09183ab38>
With this code I can´t obtain a frame.
Other options:
camera = requests.get('http://192.168.1.64', auth=('admin', contrasenia), stream=True) #INFO:requests.packages.urllib3.connectionpool:Starting new HTTP connection (1): 192.168.1.64 #DEBUG:requests.packages.urllib3.connectionpool:"GET / HTTP/1.1" 200 480 chunk = camera.iter_content(chunk_size=1024) chunk #<generator object iter_slices at 0x7fb090b7a150> bytes += bytes() #Traceback (most recent call last): # File "<stdin>", line 1, in <module> #TypeError: unsupported operand type(s) for +=: 'type' and 'bytes'
Other:
stream = urllib.request.urlopen("http://192.168.1.64") #<http.client.HTTPResponse object at 0x7fb090b82320>
Other:
out_cv2 = cv2.VideoCapture('http://192.168.1.64') #<VideoCapture 0x7fb090b6dc10> cv2.imshow(out_cv2) #Traceback (most recent call last): # File "<stdin>", line 1, in <module> #TypeError: Required argument 'mat' (pos 2) not found ret, frame = out_cv2.read() cv2.imshow('out', frame) #OpenCV Error: Assertion failed (size.width>0 && size.height>0) in imshow, file /io/opencv/modules/highgui/src/window.cpp, line 304 #Traceback (most recent call last): # File "<stdin>", line 1, in <module> #cv2.error: /io/opencv/modules/highgui/src/window.cpp:304: error: (-215) size.width>0 && size.height>0 in function imshow ret #False frame #
Other:
from requests.auth import HTTPBasicAuth requ = requests.get('http://192.168.1.64', auth=HTTPBasicAuth('admin', contrasenia), stream=True) out_file = open('img.png', 'wb') shutil.copyfileobj(requ.raw, out_file) #The file is empty
This code is from other post with similar problem but I can't use it to get my objetive. What can I do?
-
Lingchao Cao almost 6 yearsThank you so much! It saved my day!!
-
rizerphe almost 4 yearsWhile this code may solve the question, including an explanation of how and why this solves the problem would really help to improve the quality of your post, and probably result in more up-votes. Remember that you are answering the question for readers in the future, not just the person asking now. Please edit your answer to add explanations and give an indication of what limitations and assumptions apply.