Get iteration index from List.map()
Solution 1
To get access to index, you need to convert your list to a map using the asMap operator.
Example
final fruitList = ['apple', 'orange', 'mango'];
final fruitMap = fruitList.asMap(); // {0: 'apple', 1: 'orange', 2: 'mango'}
// To access 'orange' use the index 1.
final myFruit = fruitMap[1] // 'orange'
// To convert back to list
final fruitListAgain = fruitMap.values.toList();
Your Code
userBoard.asMap().map((i, element) => MapEntry(i, Stack(
GestureDetector(onTap: () {
setState(() {
// print("element=${element.toString()}");
// print("element=${userBoard[i].toString()}");
});
}),
))).values.toList();
References to other answers
- I like this answer better. Please take a look.
- If you want in multiple places try extending like this.
- Alternatively, you could also try the dart collection approach.
Solution 2
You can get index use list.indexOf
when the list has no duplicate elements。
Example
userBoard.map((element) {
// get index
var index = userBoard.indexOf(element);
return Container(
);
}).toList()
Solution 3
The easiest approach if you want to iterate
We can extend Iterable
with a new function:
import 'dart:core';
extension IndexedIterable<E> on Iterable<E> {
Iterable<T> mapIndexed<T>(T Function(E e, int i) f) {
var i = 0;
return map((e) => f(e, i++));
}
}
Usage:
myList.mapIndexed((element, index) {});
Taken from here.
Solution 4
Dart has released the collection
package that comes with a mapIndexed
extension to all Iterables.
import 'package:collection/collection.dart';
void main() {
final fruitList = ['apple', 'orange', 'mango'];
final withIndices = fruitList.mapIndexed((index, fruit) => "$index - $fruit");
print(withIndices);
}
(DartPad)
The package comes with all sorts of handy extensions and useful tools to work with collections.
Solution 5
Another approach using List.generate
:
var list = ['y', 'e', 's'];
var widgets = List.generate(list.length, (i) => Text(list[i]));
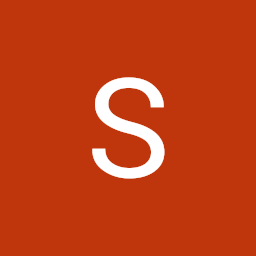
Shoham yetzhak
Updated on December 14, 2021Comments
-
Shoham yetzhak over 2 years
I wrote an iteration on list of letters and put inside cards on screen using "map" class.
In the code you can see that I made a row, and using "map" printed all the userBoard on cards to the screen. I want to add some logic inside so I need to get the id of the element (for taping event). Is there a way that I can do that?
Actually, I want to get a specific index of element over userBoard.
Code:
Widget build(BuildContext context) { return Row( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: <Widget>[ Row( children: userBoard .map((element) => Stack(children: <Widget>[ Align( alignment: Alignment(0, -0.6), child: GestureDetector( onTap: (() { setState(() { // print("element=${element.toString()}"); // print("element=${userBoard[element]}"); }); }), child: SizedBox( width: 40, height: 60, child: Card( shape: RoundedRectangleBorder( borderRadius: BorderRadius.circular(5.0), ), child: Center( child: Text(element, style: TextStyle(fontSize: 30)), )), ), ), ) ])) .toList(), ) ], ), }
Picture - each card is "element" of the map. I want to get the indexes for the function onTap.
-
Shoham yetzhak about 5 yearsI want to send a index of "userBoard" as a parameter userBoard.map((element) => Container( child: Text(element) //.... Some code and listener of button func(element_index);
-
Gökberk Yağcı almost 5 yearsIt will be wrong when dublicate element in your list it returns first founded item's index.
-
jamesdlin over 4 yearsThis would be very inefficient.
-
Henok over 4 yearsdublicate elements must be checked for sure
-
Simple UX Apps over 4 years@jamesdlin Why is it inefficient?
-
jamesdlin over 4 years@SimpleUXApps Because
list.indexOf
must iterate over the list to find the specified element. Eachlist.indexOf
call is O(n), and using it for each element of the list therefore would be O(n^2). This is inefficient since this operation could be easily done in O(n) time. -
geisterfurz007 almost 3 yearsThis is inefficient. The default implementation iterates over the list to find the given item so you have O(n²) (if I am not completely off) for this in worst case. It's also inaccurate. Given
final myList = [0, 1, 2, 1];
, this will print0, 1, 2, 1
instead of0, 1, 2, 3
. -
NearHuscarl over 2 yearsWhere is the index?
-
CopsOnRoad over 2 years@NearHuscarl This may not directly answer what is asked in the title.
-
Jani over 2 yearsA more up-to-date solution from the linked answer: "You can use the mapIndexed or forEachIndexed extension methods from the collection package."
-
Jani over 2 yearsThis should be the new accepted answer. I love how I always have to read the latest answers in Dart-related questions since the team keeps pushing out quality of life changes so fast.
-
jsgalarraga about 2 yearsThere's a much better approach from other answer here after dart released the collection package: stackoverflow.com/a/68357576/8494794
-
Amsakanna almost 2 yearsI like this better. Lemme add a link to this in my answer.
-
arg20 almost 2 yearsplease do not do this