Get Map<String, dynamic> from Map<dynamic, dynamic> flutter
Solution 1
This recent Firecast video solved my problem at 27min.
I also had an non explicit Flutter error about another nested HashMap
in my data model object that could not be converted directly.
The solution is to use Map<String, dynamic>.from(snapshot.value)
and this for every nested Map
you have in your data model object
Solution 2
I tested your code using DartPad:
void main() {
//Custom json
Map<dynamic, dynamic> json = {
"window": {
"title": "Sample Konfabulator Widget",
"name": "main_window",
"width": 500,
"height": 500
}
};
Map<dynamic, dynamic> result = json;
Map<String, dynamic> data = Map<String, dynamic>();
for (dynamic type in result.keys) {
data[type.toString()] = result[type];
}
print(data);
print(data['window']);
print(data['window']['title']);
}
Print 1:
{window: {title: Sample Konfabulator Widget, name: main_window, width: 500, height: 500}}
Print 2:
{title: Sample Konfabulator Widget, name: main_window, width: 500, height: 500}
Print 3:
Sample Konfabulator Widget
I don't understand the problem
Solution 3
This will recursively convert a Map<dynamic, dynamic> to Map<String, dynamic>:
Map<String, dynamic> dynamicMapToString(Map<dynamic, dynamic> data) {
List<dynamic> _convertList(List<dynamic> src) {
List<dynamic> dst = [];
for (int i = 0; i < src.length; ++i) {
if (src[i] is Map<dynamic, dynamic>) {
dst.add(dynamicMapToString(src[i]));
} else if (src[i] is List<dynamic>) {
dst.add(_convertList(src[i]));
} else {
dst.add(src[i]);
}
}
return dst;
}
Map<String, dynamic> retval = {};
for (dynamic key in data.keys) {
if (data[key] is Map<dynamic, dynamic>) {
retval[key.toString()] = dynamicMapToString(data[key]);
} else if (data[key] is List<dynamic>) {
retval[key.toString()] = _convertList(data[key]);
} else {
retval[key.toString()] = data[key];
}
}
return retval;
}
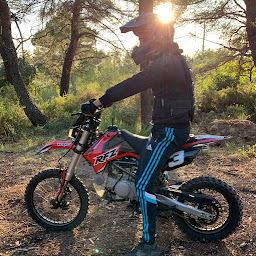
Tom3652
Updated on December 31, 2022Comments
-
Tom3652 over 1 year
I have the code below :
Map<dynamic, dynamic> result = snapshot.value; Map<String, dynamic> data = Map<String, dynamic>(); for (dynamic type in result.keys) { data[type.toString()] = result[type]; } print(data); print(data.runtimeType);
but data type is
_InternalLinkedHashMap<String, dynamic>
and i am not able to read its value, despite the ugly hack i have done above.The direct cast also doesn't work :
snapshot.value as Map<String, dynamic>
throws the error :'_InternalLinkedHashMap<Object?, Object?>' is not a subtype of type 'Map<String, dynamic>'
I need to have a Map<String, dynamic> type to be able to create my custom class Object.
snapshot.value
has a type of dynamic, but it's a json object that returns the result of a Realtime Database query and there is no documentation about how to retrieve the value into a Flutter object.I have tried this answer but i can't use it as
jsonDecode()
takes a String as a parameter.