Get month name from Date
Solution 1
Shorter version:
const monthNames = ["January", "February", "March", "April", "May", "June",
"July", "August", "September", "October", "November", "December"
];
const d = new Date();
document.write("The current month is " + monthNames[d.getMonth()]);
Note (2019-03-08) - This answer by me which I originally wrote in 2009 is outdated. See David Storey's answer for a better solution.
Solution 2
It is now possible to do this with the ECMAScript Internationalization API:
const date = new Date(2009, 10, 10); // 2009-11-10
const month = date.toLocaleString('default', { month: 'long' });
console.log(month);
'long'
uses the full name of the month, 'short'
for the short name, and 'narrow'
for a more minimal version, such as the first letter in alphabetical languages.
You can change the locale from browser's 'default'
to any that you please (e.g. 'en-us'
), and it will use the right name for that language/country.
With toLocaleString
api you have to pass in the locale and options each time. If you are going do use the same locale info and formatting options on multiple different dates, you can use Intl.DateTimeFormat
instead:
const formatter = new Intl.DateTimeFormat('fr', { month: 'short' });
const month1 = formatter.format(new Date());
const month2 = formatter.format(new Date(2003, 5, 12));
console.log(`${month1} and ${month2}`); // current month in French and "juin".
For more information see my blog post on the Internationalization API.
Solution 3
Here's another one, with support for localization :)
Date.prototype.getMonthName = function(lang) {
lang = lang && (lang in Date.locale) ? lang : 'en';
return Date.locale[lang].month_names[this.getMonth()];
};
Date.prototype.getMonthNameShort = function(lang) {
lang = lang && (lang in Date.locale) ? lang : 'en';
return Date.locale[lang].month_names_short[this.getMonth()];
};
Date.locale = {
en: {
month_names: ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December'],
month_names_short: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec']
}
};
you can then easily add support for other languages:
Date.locale.fr = {month_names: [...]};
Solution 4
I heartily recommend the format
function from, the moment.js library, which you can use like this:
moment().format("MMM"); // "Apr" - current date
moment(new Date(2012, 01, 04)).format("MMM"); // "Feb" - from a local date
moment.utc(new Date(2012, 00, 04).format("MMM"); // "Jan" - from a UTC date
Use "MMMM" instead of "MMM" if you need the full name of the month
In addition to a lengthy list of other features, it has strong support for internationalization.
Solution 5
If you don't mind extending the Date prototype (and there are some good reasons to not want to do this), you can actually come up with a very easy method:
Date.prototype.monthNames = [
"January", "February", "March",
"April", "May", "June",
"July", "August", "September",
"October", "November", "December"
];
Date.prototype.getMonthName = function() {
return this.monthNames[this.getMonth()];
};
Date.prototype.getShortMonthName = function () {
return this.getMonthName().substr(0, 3);
};
// usage:
var d = new Date();
alert(d.getMonthName()); // "October"
alert(d.getShortMonthName()); // "Oct"
These functions will then apply to all javascript Date objects.
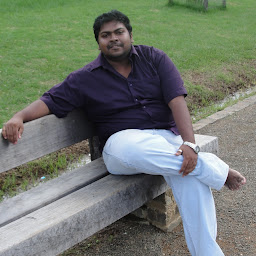
Dushan Perera
❤️ building super 🚅 fast 🏎️ web experience 🚀 Azure functions <⚡> is my recent area of interest. Former ASP.NET MVP Please feel free to suggest improvements to my posts
Updated on February 13, 2022Comments
-
Dushan Perera about 2 years
How can I generate the name of the month (e.g: Oct/October) from this date object in JavaScript?
var objDate = new Date("10/11/2009");