Get number of weekends between two dates in SQL
17,078
Solution 1
Try replacing the if statement with this:
If ((datepart(dw, @dFrom) = 1) OR (datepart(dw, @dFrom) = 7))
You should also check the end of the week to get the result.
Solution 2
I tried out this logic with several edge cases and it seems to work.
SELECT DATEDIFF(d, @dFrom, @dTo)/7+1
+ CASE WHEN DATEPART(dw,@dFrom) IN (1,7) THEN -1 ELSE 0 END
+ CASE WHEN DATEPART(dw,@dTo) IN (1,7) THEN -1 ELSE 0 END
You can change the CASE statements depending on how you want to handle cases where the start or end date is in a weekend. In my case I'm not including the weekend if the start or end date is a Saturday or Sunday.
Solution 3
DECLARE @date_from DATETIME,
@date_to DATETIME
/*TEMPORARY TABLE*/
DECLARE @DATES AS TABLE (
GDate DATETIME
)
SELECT @date_from ='2019-09-10'
SELECT @date_to ='2019-10-10'
/*DATE GENERATED*/
WITH dates
AS (
SELECT @date_from AS dt
UNION ALL
SELECT DATEADD(D, 1, dt)
FROM dates
WHERE dt < @date_to
)
/*INSERTED DATES INTO TEMP TABLE */
INSERT INTO @DATES
SELECT CONVERT(DATETIME, dt) AS Gdate FROM dates
/*Get Records from temp table*/
SELECT Gdate FROM @DATES
Related videos on Youtube
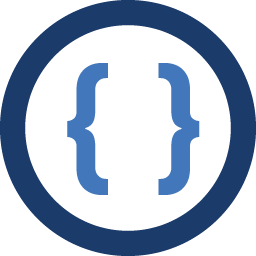
Author by
Admin
Updated on September 18, 2022Comments
-
Admin over 1 year
I need to get the number of weekends between dates in sql as a function. I have tried but stuck up somewhere in the logic.
CREATE FUNCTION fnc_NumberOfWeekEnds(@dFrom DATETIME, @dTo DATETIME) RETURNS INT AS BEGIN Declare @weekends int Set @weekends = 0 While @dFrom <= @dTo Begin If ((datepart(dw, @dFrom) = 1)) Set @weekends = @weekends + 1 Set @dFrom = DateAdd(d, 1, @dFrom) End Return (@weekends) END