Get parent directory name for a particular file using DOS Batch scripting
Solution 1
see this question
@echo OFF set mydir="%~p1" SET mydir=%mydir:\=;% for /F "tokens=* delims=;" %%i IN (%mydir%) DO call :LAST_FOLDER %%i goto :EOF :LAST_FOLDER if "%1"=="" ( @echo %LAST% goto :EOF ) set LAST=%1 SHIFT goto :LAST_FOLDER
Solution 2
It can be very simple to get the parent folder of the batch file:
@echo off
for %%a in ("%~dp0\.") do set "parent=%%~nxa"
echo %parent%
And for a parent of a file path as per the question:
@echo off
for %%a in ("c:\test\pack\a.txt") do for %%b in ("%%~dpa\.") do set "parent=%%~nxb"
echo %parent%
Solution 3
First answer above does not work if parent directory name contains a space. The following works:
@echo off
setlocal
set ParentDir=%~p1
set ParentDir=%ParentDir: =:%
set ParentDir=%ParentDir:\= %
call :getparentdir %ParentDir%
set ParentDir=%ParentDir::= %
echo ParentDir is %ParentDir%
goto :EOF
:getparentdir
if "%~1" EQU "" goto :EOF
Set ParentDir=%~1
shift
goto :getparentdir
Calling the above with parameter of "C:\Temp\Parent Dir With Space\myfile.txt" gives following:
>GetParentDir "C:\Temp\Parent Dir With Space\myfile.txt"
ParentDir is Parent Dir With Space
The above works by replacing spaces with colons (these should not exist in Windows paths), then replacing directory delimiters with spaces to so individual directories are passed to getparentdir as separate arguments. Function getparentdir loops until it finds its last argument. Finally any colons in result are replaced by spaces.
Solution 4
you can use a vbscript, eg save the below as getpath.vbs
Set objFS = CreateObject("Scripting.FileSystemObject")
Set objArgs = WScript.Arguments
strFile = objArgs(0)
WScript.Echo objFS.GetParentFolderName(strFile)
then on command line or in your batch, do this
C:\test>cscript //nologo getpath.vbs c:\test\pack\a.txt
c:\test\pack
If you want a batch method, you can look at for /?
.
%~fI - expands %I to a fully qualified path name
%~dI - expands %I to a drive letter only
%~pI - expands %I to a path only
Solution 5
Here's a way that does not use CALL
and I believe is faster.Based on jeb's splitting function (might fail if the directory name contains !
) :
@echo off
set "mydir=%~p1"
SET mydir=%mydir:~0,-1%
setlocal EnableDelayedExpansion
set LF=^
rem ** Two empty lines are required
for %%L in ("!LF!") DO (
set "dir_name=!mydir:\=%%L!"
)
for /f "delims=" %%P in (""!dir_name!"") do set "dn=%%~P"
echo %dn%
exit /b 0
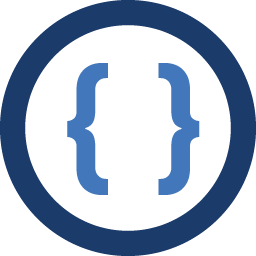
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I need to find the name of the parent directory for a file in DOS
for ex.
Suppose this is the directory
C:\test\pack\a.txt
I have a script which asks me the file name
C:\\>getname.bat enter file name: c:\test\pack\a.txt
now the script should return just the parent name of the file.
pack
and NOT the entire parent path to the file.
c:\test\pack