Get selected row in UIPickerView for each component
57,537
Solution 1
So, in your button action method, you can do something like this:
- (IBAction) showAlert {
NSUInteger numComponents = [[myPickerView datasource] numberOfComponentsInPickerView:myPickerView];
NSMutableString * text = [NSMutableString string];
for(NSUInteger i = 0; i < numComponents; ++i) {
NSUInteger selectedRow = [myPickerView selectedRowInComponent:i];
NSString * title = [[myPickerView delegate] pickerView:myPickerView titleForRow:selectedRow forComponent:i];
[text appendFormat:@"Selected item \"%@\" in component %lu\n", title, i];
}
NSLog(@"%@", text);
}
This would be the absolute formal way to retrieve information (by using the proper datasource and delegate methods), but it might be easier (depending on your set up), to just grab the selected row and then pull the information out of your model directly, instead of going through the delegate method.
Solution 2
Swift 3 version:
var value = ""
for i in 0..<numberOfComponents {
let selectedRow = pickerView.selectedRow(inComponent: i)
if let s = pickerView.delegate?.pickerView!(pickerView, titleForRow: selectedRow, forComponent: i) {
value += s
}
}
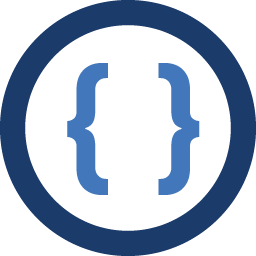
Author by
Admin
Updated on February 06, 2020Comments
-
Admin over 4 years
I have an
UIPickerView
with 3 components populated with 2NSMutableArrays
(2 components have the same array).A tutorial says:
//PickerViewController.m - (void)pickerView:(UIPickerView *)thePickerView didSelectRow:(NSInteger)row inComponent:(NSInteger)component { NSLog(@"Selected Color: %@. Index of selected color: %i", [arrayColors objectAtIndex:row], row); }
But I want to show the selected row for each component in an
UIAlertView
after the user touched an UIButton.Is there a way to do this? Or must I just use 3 invisible
UILabels
as buffer?Thanks in advance.
-
Admin almost 15 yearsThanks! selectedRowInComponent is just what I needed.
-
datwelk over 11 yearsUhm, why are you using an unsigned integer here? -[UIPickerView selectedRowInComponent:] can also return -1 if there's no selected row.