Get the sum of digits in PHP
46,138
Solution 1
array_sum(str_split($number));
This assumes the number is positive (or, more accurately, that the conversion of $number
into a string generates only digits).
Solution 2
Artefactos method is obviously unbeatable, but here an version how one could do it "manually":
$number = 1234567890;
$sum = 0;
do {
$sum += $number % 10;
}
while ($number = (int) $number / 10);
This is actually faster than Artefactos method (at least for 1234567890
), because it saves two function calls.
Solution 3
Another way, not so fast, not single line simple
<?php
$n = 123;
$nstr = $n . "";
$sum = 0;
for ($i = 0; $i < strlen($nstr); ++$i)
{
$sum += $nstr[$i];
}
echo $sum;
?>
It also assumes the number is positive.
Solution 4
function addDigits($num) { if ($num % 9 == 0 && $num > 0) { return 9; } else { return $num % 9; } }
only O(n)
at LeetCode submit result: Runtime: 4 ms, faster than 92.86% of PHP online submissions for Add Digits. Memory Usage: 14.3 MB, less than 100.00% of PHP online submissions for Add Digits.
Solution 5
<?php
// PHP program to calculate the sum of digits
function sum($num) {
$sum = 0;
for ($i = 0; $i < strlen($num); $i++){
$sum += $num[$i];
}
return $sum;
}
// Driver Code
$num = "925";
echo sum($num);
?>
Result will be 9+2+5 = 16
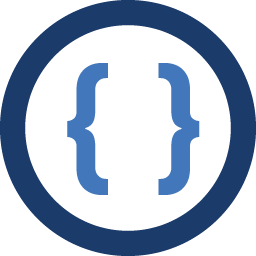
Author by
Admin
Updated on April 21, 2020Comments
-
Admin about 4 years
How do I find the sum of all the digits in a number in PHP?