Get value of registry key C#
Solution 1
I believe you want root.GetSubKeyNames()
in the loop not GetValueNames()
While values is working to get the values I would suggest the following loop:
foreach(string keyname in root.GetSubKeyNames())
{
// use key to get value and set textbox4
using (RegistryKey key = root.OpenSubKey(keyname))
{
MessageBox.Show(key.ValueCount.ToString());
}
}
Solution 2
The OpenSubKey
method does not throw an exception if the specified subkey is not found. Instead, it simply returns null
. It's your responsibility as a programmer to ensure that the appropriate key was found and opened by checking the return value of the method call.
Thus, my suspicion is that the registry key that you've specified is invalid. Open up Registry Editor (regedt32.exe
), and verify that you can find the key in the registry exactly as written.
If you find that the registry key is indeed located exactly where you thought it was, then the problem may be related to the WOW64 subsystem, which allows 64-bit versions of Windows to run 64-bit apps. If the value was written to the registry by a 32-bit program, you won't be able to read it with the above code from a 64-bit program (or vice versa). The simplest way to check this is to change the compilation settings for your project. For example, if you're currently compiling for x86, then change to compiling for x64, or vice versa. Registry redirection may also be getting in your way; this will check for that as well.
Solution 3
I wanted the very same thing and your code helped me, but as you said, it didn't work properly. So, I made some modifications and I think it works fine now! Try this:
//Just make the reference until "custom_subkey", not to the next one ("custom value")
string reg_subKey = "Software\\Custom_Subkey";
RegistryKey root = Registry.CurrentUser.CreateSubKey(reg_subKey);
//Use GetSubKeyNames, instead of GetValueNames, because now you are in a higher level
foreach (string keyname in root.GetSubKeyNames())
{
using (RegistryKey key = root.OpenSubKey(keyname))
{
foreach (string valueName in key.GetValueNames())
{
MessageBox.Show(valueName);
MessageBox.Show(key.GetValue(valueName).ToString() );
}
}
}
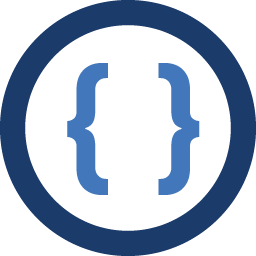
Admin
Updated on June 19, 2022Comments
-
Admin almost 2 years
I have already looked at existing topics, so please try to refrain from dropping links here.
I want to get the value of a registry key - plain and simple. Here is what I have so far.
Registry: 1) Made a key under
Current_User\Software\Custom_Subkey\Custom_Value\Custom_key\string_value
I am trying to find the string_value
string reg_subKey = "Software\\Custom_Subkey\\Custom_Value"; RegistryKey root = Registry.CurrentUser.CreateSubKey(reg_subKey); foreach (string keyname in root.GetValueNames()) { textBox4.AppendText(keyname.ToString() + Environment.NewLine); // Appends the following data to textBox4 once the foreach is completed: // Header1 // Header2 // Header3 // Header4 // Header5 // Now I want to get the VALUES of each header: using (RegistryKey key = root.OpenSubKey(keyname)) { **// THIS LINE GETS HIGHLIGHTED WITH THE FOLLOWING ERROR: "Object reference not set to an instance of an object.**" MessageBox.Show(key.ValueCount.ToString()); } }
Hopefully this is a simple fix. I look forward to hearing your responses. Thanks, Evan
-
Admin almost 13 yearsthe GetValueNames() is working fine - it's getting all of the values for the given subkey. But I then want to find the string value of the values found above. This is where I'm running into problems. I am 99.99 percent sure that the name is written exactly as it's found in registry. It seems I'll have to do some more investigating, though.
-
Hogan almost 13 yearsThe value is not the same as the key. See code sample above.
-
Admin almost 13 yearsI know this makes me sound like an ***hole but feel free to just decline... Would you be willing to make a registry key under "HKCU\software" and then add another key under this key, and then ... give this final key a value? Once the keys are there you might be able to see where I am messing up? I don't think that my OS is the issue as I'm doing this all on one machine right now (32 bit).
-
Cody Gray almost 13 years@Evan: Darn, I thought the 64-bit suggestion would be the answer. I suppose I can do that... Give me a minute.
-
Admin almost 13 yearshit the nail on the head. + 1 / Correct answer.
-
Cody Gray almost 13 years@Evan: The problem is that
root
doesn't have any subkeys! It has one value, but no subkeys. -
Admin almost 13 yearsSo would you suggest I use the provided by Hogan? It gets the value just fine, but it does not return the key name. Something I can surely modify to work but - just asking.
-
Cody Gray almost 13 years@Evan: I have no idea. It's hard to tell what you're trying to do. I think you're confusing the terminology value and key. If the code does what you want, then use it.