getting a files from remote path to local dir using sftp in python
17,805
The problem is that sftp.get()
is intended to transfer a single file not a directory. So to download entire directory you should get list of files in it and download them separately:
def download_dir(remote_dir, local_dir):
import os
os.path.exists(local_dir) or os.makedirs(local_dir)
dir_items = sftp.listdir_attr(remote_dir)
for item in dir_items:
# assuming the local system is Windows and the remote system is Linux
# os.path.join won't help here, so construct remote_path manually
remote_path = remote_dir + '/' + item.filename
local_path = os.path.join(local_dir, item.filename)
if S_ISDIR(item.st_mode):
download_dir(remote_path, local_path)
else:
sftp.get(remote_path, local_path)
download_dir("/home","C:\\Users\\ShareM\\Desktop")
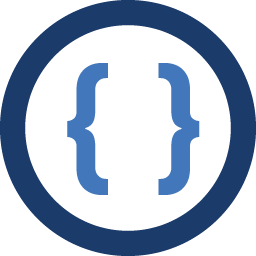
Author by
Admin
Updated on June 26, 2022Comments
-
Admin almost 2 years
I am trying to get files from remote path to my local dir. When i am executing the code i am getting an error. as described below.
import paramiko import SSHLibrary from stat import S_ISDIR server, username, password = ('Remote ID', 'root', 'root') ssh = paramiko.SSHClient() paramiko.util.log_to_file("ssh.log") ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) ssh.connect(server, username=username, password=password) ssh_stdin, ssh_stdout, ssh_stderr = ssh.exec_command('ls') print "output", ssh_stdout.read() #Reading output of the executed command error = ssh_stderr.read() #Transfering files to and from the remote machine sftp = ssh.open_sftp() print sftp.getcwd() print sftp.get_channel() print sftp.listdir("/home") sftp.get("/home","C:\\Users\\ShareM\\Desktop") #---> facing problem here sftp.close() ssh.close()
Error:-
Traceback (most recent call last): File "C:\Users\ShareM\Desktop\Automotive\devlopment\sshtesting\src\sshtest.py", line 36, in <module> sftp.get("/home","C:\\Users\\ShareM\\Desktop") File "build\bdist.win32\egg\paramiko\sftp_client.py", line 637, in get IOError: [Errno 13] Permission denied: 'C:\\Users\\ShareM\\Desktop'
Need some help.
-
Meric Ozcan almost 6 yearsso if we have a directory full of different files and folders, we need to list them all and create them in our local path. dude!
-
praveen about 5 yearswhat does the S_ISDIR(item.st_mode) mean??
-
praveen about 5 yearsfrom stat import S_ISDIR add this import to run this method.
-
ingyhere almost 4 years@praveen : It means it's a directory.
-
ingyhere almost 4 yearsAlso, it's important to separate the dir listing/stat from the actual file transfers. Some FTP servers will dump the connection unexpectedly or with too many operations.