Getting and setting class attributes in Dart / Flutter
1,042
In you code sample, you defined age
, height
, and weight
as public. So, you don't need to define getters and setters. You can directly increment and decrement their values.
void main() {
final a = Person(age: 25, height: 210, weight: 90);
a.age++;
a.height++;
a.weight++;
print(a);
// This person is 26 years old, 211cm tall and weights 91kg
}
class Person {
int age;
int height;
int weight;
Person({
this.age = 0,
this.height = 0,
this.weight = 0,
});
toString() => 'This person is $age years old, ${height}cm tall and weights ${weight}kg';
}
However, you might encounter problems by mutating objects if later, you rely on state equality to rebuild a Widget. It's better to handle your State objects as being immutable.
Then, regarding listing the values, if you refer to [dart:mirrors][1]
, it's not supported by Flutter.
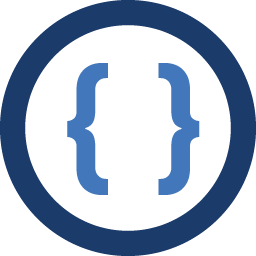
Author by
Admin
Updated on December 19, 2022Comments
-
Admin over 1 year
I would like to list a class's attributes and set them too. For example in a ListView when I tap on the value of an attribute I would like to increment it by 1.
Sample class:
class Person { int age; int height; int weight; Person({ this.age, this.height, this.weight, }); }
Is it possible to list and set these values without writing getter and setter functions for each of the attributes?