Getting checked rows from kendo grid
Solution 1
To get just the checked boxes, I use this
var grid = $("#Grid").data("kendoGrid");
grid.tbody.find("input:checked").closest("tr").each(function (index) {
whatever you need done.
});
Solution 2
The accepted answer was correct in 2014 but now the api has improved. You can use the following snippet to get the data items. Note that checked rows will also be selected and thus be returned from the grid.select() call.
//get the grid
var grid = $("#grid").data("kendoGrid");
// array to store the dataItems
var selected = [];
//get all selected rows (those which have the checkbox checked)
grid.select().each(function(){
//push the dataItem into the array
selected.push(grid.dataItem(this));
});
Solution 3
You could store the selected items by uid
, then get them from the data source when needed.
Select handler:
function selectRow() {
var checked = this.checked,
row = $(this).closest("tr"),
grid = $("#grid").data("kendoGrid"),
dataItem = grid.dataItem(row);
checkedrows[dataItem.uid] = checked;
}
To get the serialized array of items:
function getSerializedSelectedRows() {
var items = [],
item,
grid = $("#grid").data("kendoGrid");
for (var uid in checkedrows) {
if (checkedrows.hasOwnProperty(uid)) {
if (checkedrows[uid]) {
item = grid.dataSource.getByUid(uid);
items.push(item);
}
}
}
return JSON.stringify(items);
}
(demo)
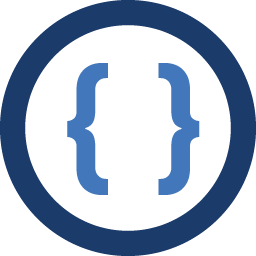
Admin
Updated on April 25, 2020Comments
-
Admin about 4 years
I have a kendo grid with a checked box column .I have been trying to get the dataitem rows when the corresponding check boxes are checked.On click of a button , I need to get only the checked rows in JSon .Here is a JSfiddle I have been playing with.It gets only the Id rather than the row data.I have been trying to modify so that it can return the entire row data .
var crudServiceBaseUrl = "http://demos.kendoui.com/service", dataSource = new kendo.data.DataSource({ transport: { read: { url: crudServiceBaseUrl + "/Products", dataType: "jsonp" }, update: { url: crudServiceBaseUrl + "/Products/Update", dataType: "jsonp" }, destroy: { url: crudServiceBaseUrl + "/Products/Destroy", dataType: "jsonp" }, create: { url: crudServiceBaseUrl + "/Products/Create", dataType: "jsonp" }, parameterMap: function (options, operation) { if (operation !== "read" && options.models) { return { models: kendo.stringify(options.models) }; } } }, batch: true, pageSize: 20, schema: { model: { id: "ProductID", fields: { ProductID: { editable: false, nullable: true }, ProductName: { validation: { required: true } }, UnitPrice: { type: "number", validation: { required: true, min: 1 } }, Discontinued: { type: "boolean" }, UnitsInStock: { type: "number", validation: { min: 0, required: true } } } } } }); //Grid definition var grid = $("#grid").kendoGrid({ dataSource: dataSource, pageable: true, height: 430, //define dataBound event handler toolbar: ["create"], columns: [ //define template column with checkbox and attach click event handler { template: "<input type='checkbox' class='checkbox' />" }, "ProductName", { field: "UnitPrice", title: "Unit Price", format: "{0:c}", width: "100px" }, { field: "UnitsInStock", title: "Units In Stock", width: "100px" }, { field: "Discontinued", width: "100px" }, { command: ["edit", "destroy"], title: " ", width: "172px" } ], editable: "inline" }).data("kendoGrid"); //bind click event to the checkbox grid.table.on("change", ".checkbox" , selectRow); $("#showSelection").bind("click", function () { var items = []; for(var i in checkedrows){ if(checkedrows[i]){ items.push([i]); } } alert(JSON.stringify(itemss)); }); }); var checkedrows = {}; var itemss =[]; //on click of the checkbox: function selectRow() { var checked = this.checked, row = $(this).closest("tr"), grid = $("#grid").data("kendoGrid"), dItem = grid.dataItem(row); checkedrows[dItem.id] = checked; if (checked) { itemss.push(dItem) //-select the row } else { itemss.pop(dataItem); } }
the row get can be accessed by the dataItem ,but how to get the dataItem based on the index .Thanks.