Getting Data out of Firebase Object with Angular2, angularfire2 and Typescript
Observables
are an asynchronous data structure, meaning that you don't actually know when the data is going to be available, you have to subscribe to the Observable
and wait for the data to become available in the next
handler.
items.first().subscribe(x => console.log(x.name));
Further, an Observable
is not static, so you can't index into it as such. If you need only the first item then I would suggest instead that you extract the value and modify it with a flatMap
+ first
+ map
combination.
af.database.list('/data')
//Convert array into Observable and flatten it
.flatMap(list => list)
//Get only the first item in this list
.first()
//Transform the value
.map(({name, firebaseKey}) => ({name: "test3", firebaseKey}))
//Subscribe to the stream
.subscribe(x => console.log(x));
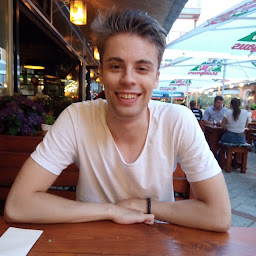
jufabeck2202
Updated on June 05, 2022Comments
-
jufabeck2202 almost 2 years
Im currently working on a Project with Angular 2 and angularFire2. My database looks like this in Firebase: base:/data
[{Name:test1}, {Name:test2}, {Name:test3}]
All of the items also have the Firebase key.
Now im trying to get data out of the Firebase with the Observabels from RX. When i do this, i get the names in the Console:
let items= af.database.list('/data').map(i=>{return i}); items.forEach(i=>i.forEach(e=>(console.log(e.name))));
Output: test1,test2,test3. Everything Works.
But when i do this:
console.log(items.first());
I get a FirebaseListObservable back.
How can i get only the First Object from the FirebaseListObservable? How can i edit Items in the list? like:
items[0].name=test3
And How can i get the Firebase Key from an Item
how can i get The firebaseKey from an Item in the list?
-
jufabeck2202 almost 8 yearsTHANK you, that really helped me.
-
Lloyd over 7 yearsWhat if you wanted to loop over the items, rather than just the first?
-
paulpdaniels over 7 years@Lloyd then you would use
flatMap
instead offirst
-
Lloyd over 7 yearsYou have flatMap in there. So just take first() out?
-
paulpdaniels over 7 yearsYes just remove the
first
in that case -
Ian Poston Framer over 6 yearsI used
af.list('/data').subscribe(x => console.log(x));
And I got all the data loaded to the app component.