Getting Error inflating class android.support.design.widget.NavigationView
Solution 1
It all start from here,
Caused by: java.lang.OutOfMemoryError: Failed to allocate a 276203532 byte allocation with 12108696 free bytes and 174MB until OOM at dalvik.system.VMRuntime.newNonMovableArray(Native Method) at android.graphics.BitmapFactory.nativeDecodeAsset(Native Method) at android.graphics.BitmapFactory.decodeStream(BitmapFactory.java:609) at android.graphics.BitmapFactory.decodeResourceStream(BitmapFactory.java:444)
Because an image you are using for the header or as elements in your navigationdrawer has a higher resolution than the application allocated memory can handle. Reduce those images sizes and check. That will resolve the issue. If you add some more stack trace below, you can find what element exactly causing this problem.
Solution 2
I got the inflater error for the Navigation view when switching to Android support library version 23.1.0, compile SDK version 23 and build tools version 23.0.2.
For me, it turned out to be a proguard issue. Add the following line to your proguard configuration:
-keep class android.support.v7.widget.LinearLayoutManager { *; }
See Android Open Source Project Issue Tracker
Solution 3
Yes, me too got same error. If you look into error carefully u will find following line Caused by: java.lang.OutOfMemoryError: Failed to allocate a 276203532 byte allocation with 12108696 free bytes and 174MB until OOM
This is can resolved by minimizing resolution of images which are used for menu.
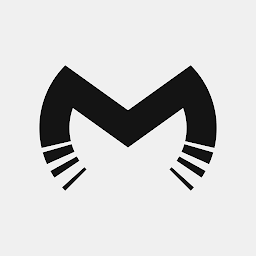
Mobin Amanzai
Updated on June 23, 2022Comments
-
Mobin Amanzai almost 2 years
I was following a tutorial on implementing the navigationView from the design support library and I just can't get away from this error below. I've read the other solutions posted on this site, but none of them worked for me, please help.
Caused by: android.view.InflateException: Binary XML file line #28: Error inflating class android.support.design.widget.NavigationView
activity_main.xml
<android.support.v4.widget.DrawerLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/drawer" android:layout_width="match_parent" android:layout_height="match_parent" android:fitsSystemWindows="true" tools:context=".MainActivity"> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <include android:id="@+id/tool_bar" layout="@layout/tool_bar" /> <FrameLayout android:id="@+id/frame" android:layout_width="match_parent" android:layout_height="match_parent"> </FrameLayout> </LinearLayout> <android.support.design.widget.NavigationView android:id="@+id/navigation_view" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_gravity="start" app:headerLayout="@layout/header" app:menu="@menu/drawer" /> </android.support.v4.widget.DrawerLayout>
MainActiviy.java
import android.support.design.widget.NavigationView; import android.support.v4.widget.DrawerLayout; import android.support.v7.app.ActionBarDrawerToggle; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.support.v7.widget.Toolbar; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.Toast; public class MainActivity extends AppCompatActivity { //Defining Variables private Toolbar toolbar; private NavigationView navigationView; private DrawerLayout drawerLayout; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // Initializing Toolbar and setting it as the actionbar toolbar = (Toolbar) findViewById(R.id.tool_bar); setSupportActionBar(toolbar); //Initializing NavigationView navigationView = (NavigationView) findViewById(R.id.navigation_view); //Setting Navigation View Item Selected Listener to handle the item click of the navigation menu navigationView.setNavigationItemSelectedListener(new NavigationView.OnNavigationItemSelectedListener() { // This method will trigger on item Click of navigation menu @Override public boolean onNavigationItemSelected(MenuItem menuItem) { //Checking if the item is in checked state or not, if not make it in checked state if (menuItem.isChecked()) menuItem.setChecked(false); else menuItem.setChecked(true); //Closing drawer on item click drawerLayout.closeDrawers(); //Check to see which item was being clicked and perform appropriate action switch (menuItem.getItemId()) { //Replacing the main content with ContentFragment Which is our Inbox View; case R.id.inbox: Toast.makeText(getApplicationContext(), "Inbox Selected", Toast.LENGTH_SHORT).show(); ContentFragment fragment = new ContentFragment(); android.support.v4.app.FragmentTransaction fragmentTransaction = getSupportFragmentManager().beginTransaction(); fragmentTransaction.replace(R.id.frame, fragment); fragmentTransaction.commit(); return true; // For rest of the options we just show a toast on click case R.id.starred: Toast.makeText(getApplicationContext(), "Stared Selected", Toast.LENGTH_SHORT).show(); return true; case R.id.sent_mail: Toast.makeText(getApplicationContext(), "Send Selected", Toast.LENGTH_SHORT).show(); return true; case R.id.drafts: Toast.makeText(getApplicationContext(), "Drafts Selected", Toast.LENGTH_SHORT).show(); return true; case R.id.allmail: Toast.makeText(getApplicationContext(), "All Mail Selected", Toast.LENGTH_SHORT).show(); return true; case R.id.trash: Toast.makeText(getApplicationContext(), "Trash Selected", Toast.LENGTH_SHORT).show(); return true; case R.id.spam: Toast.makeText(getApplicationContext(), "Spam Selected", Toast.LENGTH_SHORT).show(); return true; default: Toast.makeText(getApplicationContext(), "Somethings Wrong", Toast.LENGTH_SHORT).show(); return true; } } }); // Initializing Drawer Layout and ActionBarToggle drawerLayout = (DrawerLayout) findViewById(R.id.drawer); ActionBarDrawerToggle actionBarDrawerToggle = new ActionBarDrawerToggle(this, drawerLayout, toolbar, R.string.openDrawer, R.string.closeDrawer) { @Override public void onDrawerClosed(View drawerView) { // Code here will be triggered once the drawer closes as we dont want anything to happen so we leave this blank super.onDrawerClosed(drawerView); } @Override public void onDrawerOpened(View drawerView) { // Code here will be triggered once the drawer open as we dont want anything to happen so we leave this blank super.onDrawerOpened(drawerView); } }; //Setting the actionbarToggle to drawer layout drawerLayout.setDrawerListener(actionBarDrawerToggle); //calling sync state is necessay or else your hamburger icon wont show up actionBarDrawerToggle.syncState(); } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.menu_main, menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { // Handle action bar item clicks here. The action bar will // automatically handle clicks on the Home/Up button, so long // as you specify a parent activity in AndroidManifest.xml. int id = item.getItemId(); //noinspection SimplifiableIfStatement if (id == R.id.action_settings) { return true; } return super.onOptionsItemSelected(item); } }
build.gradle
apply plugin: 'com.android.application' android { compileSdkVersion 23 buildToolsVersion '23.0.0' defaultConfig { applicationId "com.example.mobinamanzai.projectalpha" minSdkVersion 17 targetSdkVersion 23 versionCode 1 versionName "1.0" } buildTypes { release { minifyEnabled false proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro' } debug { debuggable true } } } dependencies { compile fileTree(dir: 'libs', include: ['*.jar']) compile 'com.android.support:support-v4:23.0.0' compile 'com.android.support:appcompat-v7:23.0.0' compile 'com.android.support:design:23.0.0' compile 'de.hdodenhof:circleimageview:1.3.0' }
EDIT:
Stack Trace Caused by: android.view.InflateException: Binary XML file line #28: Error inflating class android.support.design.widget.NavigationView Caused by: java.lang.reflect.InvocationTargetException Caused by: android.view.InflateException: Binary XML file line #2: Error inflating class android.widget.RelativeLayout Caused by: java.lang.reflect.InvocationTargetException Caused by: java.lang.OutOfMemoryError: Failed to allocate a 276203532 byte allocation with 12108696 free bytes and 174MB until OOM
EDIT 2: The problem seems to be in the header.xml
-
diyoda_ over 8 yearsNo problem. Glad I could be at any help.
-
box over 8 yearsThanks man! I had the exact same issue and I don't know how would I solve it without your help :).
-
neelabh about 8 yearsFor me the Force close said Caused by: java.lang.ClassNotFoundException: android.support.v7.widget.LinearLayoutManager and thus the right fix for me
-
frostymarvelous about 8 yearsThis actually solves a different problem but still nice to have here