Getting location offline
Just use this code. Make sure the user hasn't selected wifi only or network only option in his location setings. it has to be high accuracy or GPS only. This piece of code will work.
public class Location extends AppCompatActivity {
LocationManager locationManager;
Context mContext;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_location);
mContext=this;
locationManager=(LocationManager) mContext.getSystemService(Context.LOCATION_SERVICE);
locationManager.requestLocationUpdates( LocationManager.GPS_PROVIDER,
2000,
10, locationListenerGPS);
isLocationEnabled();
}
LocationListener locationListenerGPS=new LocationListener() {
@Override
public void onLocationChanged(android.location.Location location) {
double latitude=location.getLatitude();
double longitude=location.getLongitude();
String msg="New Latitude: "+latitude + "New Longitude: "+longitude;
Toast.makeText(mContext,msg,Toast.LENGTH_LONG).show();
}
@Override
public void onStatusChanged(String provider, int status, Bundle extras) {
}
@Override
public void onProviderEnabled(String provider) {
}
@Override
public void onProviderDisabled(String provider) {
}
};
protected void onResume(){
super.onResume();
isLocationEnabled();
}
private void isLocationEnabled() {
if(!locationManager.isProviderEnabled(LocationManager.GPS_PROVIDER)){
AlertDialog.Builder alertDialog=new AlertDialog.Builder(mContext);
alertDialog.setTitle("Enable Location");
alertDialog.setMessage("Your locations setting is not enabled. Please enabled it in settings menu.");
alertDialog.setPositiveButton("Location Settings", new DialogInterface.OnClickListener(){
public void onClick(DialogInterface dialog, int which){
Intent intent=new Intent(Settings.ACTION_LOCATION_SOURCE_SETTINGS);
startActivity(intent);
}
});
alertDialog.setNegativeButton("Cancel", new DialogInterface.OnClickListener(){
public void onClick(DialogInterface dialog, int which){
dialog.cancel();
}
});
AlertDialog alert=alertDialog.create();
alert.show();
}
else{
AlertDialog.Builder alertDialog=new AlertDialog.Builder(mContext);
alertDialog.setTitle("Confirm Location");
alertDialog.setMessage("Your Location is enabled, please enjoy");
alertDialog.setNegativeButton("Back to interface",new DialogInterface.OnClickListener(){
public void onClick(DialogInterface dialog, int which){
dialog.cancel();
}
});
AlertDialog alert=alertDialog.create();
alert.show();
}
}
}
The parameters of requestLocationUpdates methods are as follows:
provider: the name of the provider with which we would like to register. minTime: minimum time interval between location updates (in milliseconds). minDistance: minimum distance between location updates (in meters). listener: a LocationListener whose onLocationChanged(Location) method will be called for each location update.
Permissions:
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
Add above permissions to manifest file for the version lower than lollipop and for marshmallow and higher version use runtime permission.
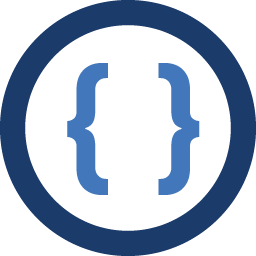
Admin
Updated on June 25, 2022Comments
-
Admin almost 2 years
I want to get the value of Longitude and Latitude of my current locatoin when offline and save the current location to its database. Is it possible to get the longitude and latitude of the device when mobile data and wifi are off but the GPS is ON?