Getting node value from XML string using javascript
12,293
The .nodeValue
property of a DOM element is always null.
Use .textContent
instead.
alert(code.textContent);
I would also suggest using DOM traversal methods that don't require sifting through each individual child node by index:
var attributes = item.getElementsByTagName("attribute"); // should contain 4 elements
See also: nodeValue vs innerHTML and textContent. How to choose?
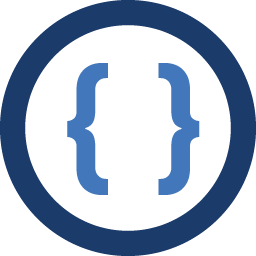
Author by
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I am trying to retrieve the 'code' and the 'value' from an XML string.
I have the following XML string:
<items> <item> <id>55</id> <attributes> <attribute> <code>ID</code> <value><![CDATA[55]]></value> </attribute> <attribute> <code>Chip_ID</code> <value><![CDATA[1]]></value> </attribute> <attribute> <code>FilterKey</code> <value><![CDATA[5]]></value> </attribute> <attribute> <code>DateTime</code> <value><![CDATA[22/12/2014 12:21:25]]></value> </attribute> </attributes> </item> </items>
I then have the following javaScript to identify each node:
var xmlDocument = new ActiveXObject('Microsoft.XMLDOM'); xmlDocument.async = false; xmlDocument.loadXML(pXML); var oFirstNode = xmlDocument.documentElement; var item = oFirstNode.childNodes[0]; //10 of these and they represent the items //alert("1 "+item.nodeName); var ID = item.childNodes[0]; //one of these for each level-ID - NO CHILDREN var attributes = item.childNodes[1]; //one of these for each level-attributes //alert("2 " + ID.nodeName); //alert("2 " + attributes.nodeName); var attribute = attributes.childNodes[0];//4 of these for each level and they all have 2 children-code and value //alert("3 " + attribute.nodeName); var code = attribute.childNodes[0]; var value = attribute.childNodes[1]; alert(code.nodeName); alert(value.nodeName);
I know I am at the correct node as the alert boxes are all giving the expected values.
I now want to retrieve the text for 'code' and 'value' eg the first entry should return code = ID value = ![CDATA[55]]
I have tried:
alert(code.nodeValue); alert(value.nodeValue);
but they are both coming back null.
-
Admin over 9 yearsstill no luck, it will not recognise getElementsByTagName
-
JLRishe over 9 years@user2970105
code.textContent
seems to be working here: jsfiddle.net/o3cp2wc5 (I'm using jQuery to parse the XML since my browser doesn't support ActiveXObject). What version of IE are you using? -
JLRishe over 9 years@user2970105 And here is an example of using
.getElementsByTagName()
to select elements: jsfiddle.net/onjs7kLm