Getting NullPointerException when trying to stream().filter().collect(Collectors.toList())) on Java list
Very likely u.name
is null
Try to change your Predicate
in
Predicate<Customer> customerPredicate = u -> (u.name != null && u.name.toLowerCase().contains(keyword.toLowerCase()));
To see what's the Customer instance that has name == null
, you could add a peek
method in your chain.
customerList.stream()
.peek(c -> {
if (c.name == null) {
// do something...
}
}).filter(customerPredicate).collect(Collectors.toList());
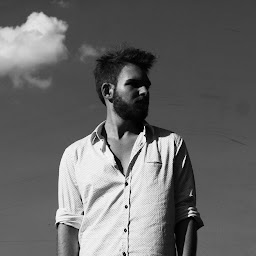
Elias Johannes
Updated on July 09, 2022Comments
-
Elias Johannes almost 2 years
I got a problem while trying to search a specific Java object in a list.
Actually i got the search function i want working for another Java class. Now i tried it for a different one but instead of returning a list of results i get a NullPointerException.This is how my function looks:
public static List<Customer> searchByEverything(String keyword){ List<Customer> customerList = find.all(); //Using java Ebean System.out.println(keyword); //Check if keyword is not empty System.out.println(customerList); //Check if list is not empty Predicate<Customer> customerPredicate = u -> u.name.toLowerCase().contains(keyword.toLowerCase()); try{ return customerList.stream().filter(customerPredicate).collect(Collectors.toList()); }catch (Exception e){ e.printStackTrace(); }
This is the strack trace from Exception e:
java.lang.NullPointerException at models.Customer.lambda$searchByEverything$2(Customer.java:174) at java.util.stream.ReferencePipeline$2$1.accept(ReferencePipeline.java:174) at java.util.ArrayList$Itr.forEachRemaining(ArrayList.java:891) at java.util.Spliterators$IteratorSpliterator.forEachRemaining(Spliterators.java:1801) at java.util.stream.AbstractPipeline.copyInto(AbstractPipeline.java:481) at java.util.stream.AbstractPipeline.wrapAndCopyInto(AbstractPipeline.java:471) at java.util.stream.ReduceOps$ReduceOp.evaluateSequential(ReduceOps.java:708) at java.util.stream.AbstractPipeline.evaluate(AbstractPipeline.java:234) at java.util.stream.ReferencePipeline.collect(ReferencePipeline.java:499) at models.Customer.searchByEverything(Customer.java:179) at controllers.Application.searchCustomers(Application.java:262) at router.Routes$$anonfun$routes$1$$anonfun$applyOrElse$17$$anonfun$apply$17.apply(Routes.scala:479) at router.Routes$$anonfun$routes$1$$anonfun$applyOrElse$17$$anonfun$apply$17.apply(Routes.scala:479) at play.core.routing.HandlerInvokerFactory$$anon$4.resultCall(HandlerInvoker.scala:157) at play.core.routing.HandlerInvokerFactory$$anon$4.resultCall(HandlerInvoker.scala:156) at play.core.routing.HandlerInvokerFactory$JavaActionInvokerFactory$$anon$14$$anon$3$$anon$1.invocation(HandlerInvoker.scala:136) at play.core.j.JavaAction$$anon$1.call(JavaAction.scala:73) at play.http.HttpRequestHandler$1.call(HttpRequestHandler.java:54) at play.mvc.Security$AuthenticatedAction.call(Security.java:53) at play.core.j.JavaAction$$anonfun$7.apply(JavaAction.scala:108) at play.core.j.JavaAction$$anonfun$7.apply(JavaAction.scala:108) at scala.concurrent.impl.Future$PromiseCompletingRunnable.liftedTree1$1(Future.scala:24) at scala.concurrent.impl.Future$PromiseCompletingRunnable.run(Future.scala:24) at play.core.j.HttpExecutionContext$$anon$2.run(HttpExecutionContext.scala:56) at play.api.libs.iteratee.Execution$trampoline$.execute(Execution.scala:70) at play.core.j.HttpExecutionContext.execute(HttpExecutionContext.scala:48) at scala.concurrent.impl.Future$.apply(Future.scala:31) at scala.concurrent.Future$.apply(Future.scala:492) at play.core.j.JavaAction.apply(JavaAction.scala:108) at play.api.mvc.Action$$anonfun$apply$2$$anonfun$apply$5$$anonfun$apply$6.apply(Action.scala:112) at play.api.mvc.Action$$anonfun$apply$2$$anonfun$apply$5$$anonfun$apply$6.apply(Action.scala:112) at play.utils.Threads$.withContextClassLoader(Threads.scala:21) at play.api.mvc.Action$$anonfun$apply$2$$anonfun$apply$5.apply(Action.scala:111) at play.api.mvc.Action$$anonfun$apply$2$$anonfun$apply$5.apply(Action.scala:110) at scala.Option.map(Option.scala:146) at play.api.mvc.Action$$anonfun$apply$2.apply(Action.scala:110) at play.api.mvc.Action$$anonfun$apply$2.apply(Action.scala:103) at scala.concurrent.Future$$anonfun$flatMap$1.apply(Future.scala:251) at scala.concurrent.Future$$anonfun$flatMap$1.apply(Future.scala:249) at scala.concurrent.impl.CallbackRunnable.run(Promise.scala:32) at akka.dispatch.BatchingExecutor$AbstractBatch.processBatch(BatchingExecutor.scala:55) at akka.dispatch.BatchingExecutor$BlockableBatch$$anonfun$run$1.apply$mcV$sp(BatchingExecutor.scala:91) at akka.dispatch.BatchingExecutor$BlockableBatch$$anonfun$run$1.apply(BatchingExecutor.scala:91) at akka.dispatch.BatchingExecutor$BlockableBatch$$anonfun$run$1.apply(BatchingExecutor.scala:91) at scala.concurrent.BlockContext$.withBlockContext(BlockContext.scala:72) at akka.dispatch.BatchingExecutor$BlockableBatch.run(BatchingExecutor.scala:90) at akka.dispatch.TaskInvocation.run(AbstractDispatcher.scala:39) at akka.dispatch.ForkJoinExecutorConfigurator$AkkaForkJoinTask.exec(AbstractDispatcher.scala:415) at scala.concurrent.forkjoin.ForkJoinTask.doExec(ForkJoinTask.java:260) at scala.concurrent.forkjoin.ForkJoinPool$WorkQueue.runTask(ForkJoinPool.java:1339) at scala.concurrent.forkjoin.ForkJoinPool.runWorker(ForkJoinPool.java:1979) at scala.concurrent.forkjoin.ForkJoinWorkerThread.run(ForkJoinWorkerThread.java:107)
As i said, i have exactly the same search implemented for a different class and it works perfectly fine. Both
customerList
andkeyword
are not empty. The only difference between searching in mycustomerList
and the other list, is that it has around 4500 objects with around 50 attributes per object. Maybe that's the problem?Thank you in advance!
-
nbokmans almost 7 yearsWhile this question will 100% get closed as duplicate of "What is a NPE" question, could you post this line:
at models.Customer.lambda$searchByEverything$2(Customer.java:174)
(so line 174) -
Alberto S. almost 7 yearsMaybe there are some users whose
name
attribute is null -
Abubakkar almost 7 yearsJust a side-note, you can also use
parallelStream
(might improve performance stackoverflow.com/documentation/java/88/streams/2785/…) -
f1sh almost 7 years@nbokmans that's the lambda itself. The lambda's code is part of the question.
-
Elias Johannes almost 7 yearsSorry if this is a duplicate... i'm really overstrained with this problem. @nbokmans As f1sh says, this line is the definition of the lambda expression.
-
-
Elias Johannes almost 7 yearsWhile i'm not getting a NullPointerException anymore, it returns all of the objects now. You are probably right that u.name is null, but i can't figure out why?
-
freedev almost 7 years@EliasJohannes I've updated my answer.
-
Elias Johannes almost 7 yearsThank you, this did the job. probably really was that there were null references in the DB.