Getting text value from a jButton
Solution 1
This behavior is not reproducible in java 1.7.0_45 or 1.7.0_25, it might be a weird occurrence of String interning for your java version.
In order for your code to work properly on all java versions you have to use equals()
==
compares objects meanwhile .equals()
compares the content of the string objects.
jButton1.getText().equals("X")
Solution 2
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
class MyFrame extends JFrame{
JButton equalsButton;
JLabel ansLabel;
JLabel addLabel;
JTextField text1;
JTextField text2;
MyFrame (){
setSize(300,300);
setDefaultCloseOperation(3);
setLayout(new FlowLayout());
text1=new JTextField(10);
add(text1);
addLabel=new JLabel("+");
add(addLabel);
text2=new JTextField(10);
add(text2);
equalsButton=new JButton("=");
equalsButton.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent evt){
int num1=Integer.parseInt(text1.getText());
int num2=Integer.parseInt(text2.getText());
int tot=num1+num2;
ansLabel.setText(Integer.toString(tot));
}
});
add(equalsButton);
ansLabel=new JLabel(" ");
add(ansLabel);
pack();
setVisible(true);
}
}
class Demo{
public static void main(String args[]){
MyFrame f1=new MyFrame();
}
}
Solution 3
This was also driving me nuts when using AWT Button class... Here's the answer: There is no .getText() method for a Button... you need to use .getLabel()
Now, the story for JButtons: Depending on your version of java, getLabel() was deprecated, and finally replaced by getText... Aren't namespaces wonderful?
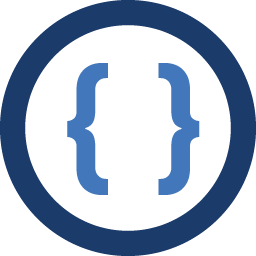
Admin
Updated on June 15, 2020Comments
-
Admin about 4 years
So I need to simply check whether a clicked button's text is
"X"
or"O"
(making tic tac toe) This code doesn't work:if (jButton1.getText()=="X")
However the following code does work:
String jButText = jButton1.getText(); if (jButText=="X")
Why doesn't the first bit of code work when the second does? Does it need to be something more like if (
jButton1.getText().toString=="X"
)? By the way, I don't thinktoString
exists in Java. That is just somewhat the equivalent in Visual Basic, which is what I normally use to create GUIs. -
spydon over 10 yearsYou wont get a NullPointerException in the last case as getText() will return an empty string.
-
Dawood ibn Kareem over 10 yearsThis doesn't answer the question. See my comment under the question.
-
Radiodef over 10 yearsInterning is what I thought first too but it doesn't explain why the first condition doesn't work. If "X" is an intern then getText returns "X" whether you assign it to a variable or not.
-
spydon over 10 yearsIt is because
jButton1.setText()
explicitly creates a new string object which is used in that comparison. -
Dawood ibn Kareem over 10 yearsI'm not convinced. OP doesn't say that he/she wrote
new String(jButton1.getText())
, so this doesn't seem to apply. It certainly doesn't explain why OP's two original snippets behave differently from each other. My honest suspicion is that OP has made a mistake understanding his/her results. -
Dawood ibn Kareem over 10 yearsIf
setText
creates a newString
object, then that still doesn't explain why the second snippet works. -
Victor Ribeiro da Silva Eloy over 10 yearsyou're rigth @spydon, my mistake. ;)
-
spydon over 10 yearsHmm you're right and I checked the source code for AbstractButton, it doesn't create a new string object on setText() it simply does
String oldValue = this.text; this.text = text;
and getText() only returns text. -
Dawood ibn Kareem over 10 yearsRight, and if that's the case, I would expect both snippets to work fine, assuming OP is doing something like
jButton1.setText("X")
to set the text in the button, because this would be in the String pool. As I said in my comment under the question, I have no idea why one snippet would work but not the other. -
spydon over 10 yearsAbstractButton declares
private String text = "";
which means that text changes when setText is called and the compiler can't treat is as a constant, but it seems like it does some "smart stuff" to jButText as it is never changed? -
spydon over 10 yearsHe must have done something wrong or have a weird java version, it is not reproducible in java 1.7.0_45.