global name 'request' is not defined
Solution 1
What you are writing is a template context processor, and you should write it like this:
def loggedin(request):
return {'username': request.session.get('username','')}
Save it in a file, and add it to TEMPLATE_CONTEXT_PROCESSORS
in your settings.py
after the 'django.core.context_processors.request',
line.
If you named the file as someapp/processors.py
, then you'll add 'somapp.processors.loggedin',
to the tuple.
Then, as long as you are returning an instance of RequestContext
, you'll have {{ username }}
in your templates.
Solution 2
Think about it. Request is not passed as an argument, therefore it is unknown in the scope of the loggedin()
function.
You can simply pass the request like
susername = loggedin(request)
Or, in this case, just pass what you need to check.
susername = loggedin(request.session)
And change the function to
def loggedin(session):
return session['username'] if session.get('username') else ''
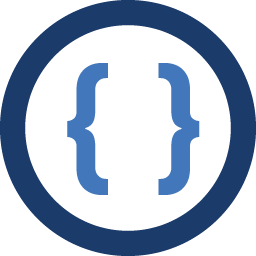
Admin
Updated on June 05, 2022Comments
-
Admin about 2 years
When using the example "views.py without functions.py", it is working fine and session is being checked alright, so it returns the username.
But, when using the second example, with the functions.py, is showing me the following error
NameError at /login/
global name 'request' is not defined
views.py without functions.py
def main(request): c = {} c.update(csrf(request)) if request.session.get('username'): susername = request.session['username'] else: susername = "" return render_to_response("login/login.html",{'username': susername},context_instance=RequestContext(request))
views.py with functions.py
def main(request): c = {} c.update(csrf(request)) susername = loggedin() return render_to_response("login/login.html",{'username': susername},context_instance=RequestContext(request))
functions.py
from django.template import RequestContext def loggedin(): if request.session.get('username'): susername = request.session['username'] else: susername = "" return susername
Why is second one not working and how can I solve it?
Thanks
-
karthikr about 11 years+1 While this is a good approach, I think the OP is trying out a simple tutorial, in which he just forgot to send the request object as a parameter.
-
Achrome about 11 yearsFixed. Thanks @Alasdair
-
Alasdair about 11 years
return session.get('username', '')
would be better, but at least the code will work now. -
zurfyx about 11 years+1 Wow, this what better than what I was looking for. Actually, I wondered if that would be possible in Python.