gmail smtp with rails 3
Solution 1
You don't need tlsmail
gem anymore at least with Rails 3.2
This will be sufficient
config.action_mailer.delivery_method = :smtp
config.action_mailer.smtp_settings = {
:address => "smtp.gmail.com",
:port => 587,
:domain => 'baci.lindsaar.net',
:user_name => '<username>',
:password => '<password>',
:authentication => 'plain',
:enable_starttls_auto => true }
From the all-mighty-guide ActionMailer configuration for gmail
Solution 2
add tlsmail to gemfile
gem 'tlsmail'
run :
bundle install
add these settings to config/envirnoments/development.rb file
YourApplicationName::Application.configure do
require 'tlsmail'
Net::SMTP.enable_tls(OpenSSL::SSL::VERIFY_NONE)
ActionMailer::Base.delivery_method = :smtp
ActionMailer::Base.perform_deliveries = true
ActionMailer::Base.raise_delivery_errors = true
ActionMailer::Base.smtp_settings = {
:address => "smtp.gmail.com",
:port => "587",
:domain => "gmail.com",
:enable_starttls_auto => true,
:authentication => :login,
:user_name => "<addreee>@gmail.com",
:password => "<password>"
}
config.action_mailer.raise_delivery_errors = true
Solution 3
You should check that [email protected] has actually sent the email. We have had issues with this in the past when sending verification emails out through Gmail's SMTP server, since sending in bulk end up not sending at all.
I suggest you log into [email protected] and verify that there are no problems and that the emails are sent.
If not, you may want try a service like Send Grid to send outgoing emails.
Alternatively, you can look into your server. Or if you are in development, have a look at log/development.log
. I'm pretty sure that you can see in your logs that it's actually trying to send the mail.
The problem is that Google doesn't trust your local IP address and your mails won't get delivered (not even to the spam directory). There is no way to work around this but using a white-listed server.
You can try this out by deploying your app into a production server like Heroku and test it there.
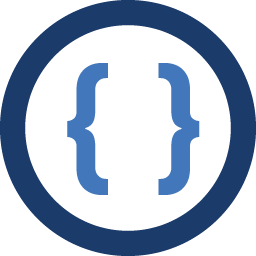
Admin
Updated on August 23, 2020Comments
-
Admin over 3 years
I am trying to get a confirmation email sending using a gmail account. I have looked around and there is nothing that is obvious. There is no errors or anything, it just dosn't send
I have this as the initalizer:
ActionMailer::Base.delivery_method = :smtp ActionMailer::Base.perform_deliveries = true ActionMailer::Base.raise_delivery_errors = true ActionMailer::Base.smtp_settings = { :address => "smtp.gmail.com", :port => 587, :domain => "gmail.com", :user_name => "<address>@gmail.com", :password => "<password>", :authentication => "plain", :enable_starttls_auto => true } ActionMailer::Base.default_url_options[:host] = "localhost:3000"