Go string to ascii byte array
64,185
Solution 1
If you're looking for a conversion, just do byteArray := []byte(myString)
The language spec details conversions between strings and certain types of arrays (byte for bytes, int for Unicode points)
Solution 2
You may not need to do anything. If you only need to read bytes of a string, you can do that directly:
c := s[3]
cthom06's answer gives you a byte slice you can manipulate:
b := []byte(s)
b[3] = c
Then you can create a new string from the modified byte slice if you like:
s = string(b)
But you mentioned ASCII. If your string is ASCII to begin with, then you are done. If it contains something else, you have more to deal with and might want to post another question with more details about your data.
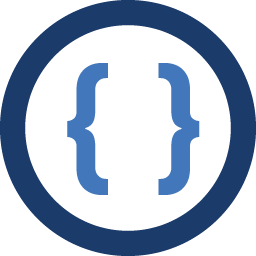
Author by
Admin
Updated on July 11, 2022Comments
-
Admin almost 2 years
How can I encode my string as ASCII byte array?
-
Edwin Biemond almost 14 yearsI think cthom06 realizes this, but this isn't, strictly speaking, an "ASCII" byte array. It's more like a UTF-8 byte array. If the string contained non-ASCII characters, then the bytes for those characters will be here too. If you want your code to play well with different languages, that's something you should always keep in mind.
-
cthom06 almost 14 years@Chickencha that's true. I kind of gave the quick and dirty answer. But i did mention the []int conversion for better unicode handling
-
hannson about 12 yearsAs of Go version 1 you should use the built-in rune datatype for Unicode handling.
-
fjjiaboming about 8 yearsThe language spec details conversions between strings and certain types of arrays (byte for bytes, int for Unicode points). => The encoding/decoding is UTF8.
-
TehSphinX over 4 yearsWhat about ASCII characters greater 127? This solution returns a 2-byte character then, but ASCII is one-byte only. For example character 170?