google apps script: html - form submit, get input values
Html form has a default behavior of navigating to the submission link. To prevent that default behavior you can use event.preventDefault()
function in HTML like so:
<form id="myForm" onsubmit="event.preventDefault(); google.script.run.processForm(this)">
Note: You can find more detailed explanation here
The form elements are sent as an object in the argument to the processForm function, to view them you can use JSON.stringfy()
. Learn more about objects here
Your processForm function would be modified like so:
function processForm(formObject) {
var ui = SpreadsheetApp.getUi();
ui.alert(JSON.stringify(formObject))
// To access individual values, you would do the following
var firstName = formObject.firstname
//based on name ="firstname" in <input type="text" name="firstname">
// Similarly
var lastName = formObject.lastname
var gender = formObject.gender
ui.alert (firstName+";"+lastName+";"+gender)
}
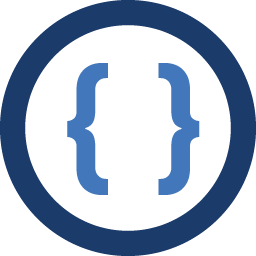
Admin
Updated on June 20, 2022Comments
-
Admin almost 2 years
I'm trying to use a sidebar with a form to get user input. The code is bound to a Google Sheets file.
Code.gs:function onOpen() { SpreadsheetApp.getUi() .createMenu('Custom Menu') .addItem('Show sidebar', 'showSidebar') .addToUi(); } function showSidebar() { var html = HtmlService.createHtmlOutputFromFile('Page') .setTitle('My custom sidebar') .setWidth(300); SpreadsheetApp.getUi() .showSidebar(html); } function processForm(formObject) { var ui = SpreadsheetApp.getUi(); ui.alert("This should show the values submitted."); }
Page.html:
<!DOCTYPE html> <html> <head> <base target="_top"> </head> <body> Please fill in the form below.<br><br> <form id="myForm" onsubmit="google.script.run.processForm(this)"> First name: <input type="text" name="firstname"><br><br> Last name: <input type="text" name="lastname"><br><br> Gender:<br> <input type="radio" name="gender" value="male" checked> Male<br> <input type="radio" name="gender" value="female"> Female<br> <input type="radio" name="gender" value="other"> Other<br> <br> <input type="submit" value="Submit"> </form><br> <input type="button" value="Cancel" onclick="google.script.host.close()" /> </body> </html>
When I press "Submit", the alert opens with the given message and my browser opens to a url which shows a blank page (https://n-ms22tssp5ubsirhhfqrplmp6jt3yg2zmob5vdaq-0lu-script.googleusercontent.com/userCodeAppPanel?firstname=&lastname=&gender=male). I'd like the alert to display the values submitted, and I don't want the extra page to open.
Pretty simple problem, but everything I read seems really over complicated. I just want to know the simplest way to get user input.