Google chrome closes immediately after being launched with selenium
Solution 1
My guess is that the driver gets garbage collected, in C++ the objects inside a function (or class) get destroyed when out of context. Python doesn´t work quite the same way but its a garbage collected language. Objects will be collected once they are no longer referenced.
To solve your problem you could pass the object reference as an argument, or return it.
def launchBrowser():
chrome_options = Options()
chrome_options.binary_location="../Google Chrome"
chrome_options.add_argument("start-maximized");
driver = webdriver.Chrome(chrome_options=chrome_options)
driver.get("http://www.google.com/")
return driver
driver = launchBrowser()
Solution 2
Just simply add:
while(True):
pass
To the end of your function. It will be like this:
def launchBrowser():
chrome_options = Options()
chrome_options.binary_location="../Google Chrome"
chrome_options.add_argument("disable-infobars");
driver = webdriver.Chrome(chrome_options=chrome_options)
driver.get("http://www.google.com/")
while(True):
pass
launchBrowser()
Solution 3
The browser is automatically disposed once the variable of the driver is out of scope. So, to avoid quitting the browser, you need to set the instance of the driver on a global variable:
Dim driver As New ChromeDriver
Private Sub Use_Chrome()
driver.Get "https://www.google.com"
' driver.Quit
End Sub
Solution 4
To make the borwser stay open I am doing this:
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
def browser_function():
driver_path = "path/to/chromedriver"
chr_options = Options()
chr_options.add_experimental_option("detach", True)
chr_driver = webdriver.Chrome(driver_path, options=chr_options)
chr_driver.get("https://target_website.com")
Solution 5
My solution is to define the driver in the init function first, then it won't close up the browser even the actional
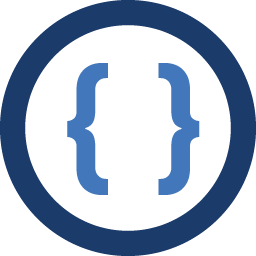
Admin
Updated on June 25, 2021Comments
-
Admin almost 3 years
I am on Mac OS X using selenium with python 3.6.3.
This code runs fine, opens google chrome and chrome stays open.:
chrome_options = Options() chrome_options.binary_location="../Google Chrome" chrome_options.add_argument("disable-infobars"); driver = webdriver.Chrome(chrome_options=chrome_options) driver.get("http://www.google.com/")
But with the code wrapped inside a function, the browser terminates immediately after opening the page:
def launchBrowser(): chrome_options = Options() chrome_options.binary_location="../Google Chrome" chrome_options.add_argument("disable-infobars"); driver = webdriver.Chrome(chrome_options=chrome_options) driver.get("http://www.google.com/") launchBrowser()
I want to use the same code inside a function while keeping the browser open.
-
d_kennetz almost 5 yearsplease add an explanation to your answer.
-
Mazhar Ali over 2 yearsBy setting driver as a global variable in init method worked.
-
Ice Bear over 2 yearsThis answer should be checked! +1 up vote for explaining the garbage concept. Clearly it's the main cause of it
-
Meet over 2 yearsHi. Will this concept still work if I close the python program? In my case, I am opening a webpage using a python script. Once the page is loaded and there are no errors, I want the python program to end. But I do not want to close the browser. I want to continue working on the browser manually. Can we do that?
-
Petar Petrov about 2 years@Meet I expect weird things to happen that way. You could instead spawn your browser with the debug port open, and connect your webdriver to it.