Google PlacePicker Closes Immediately After Launch
Solution 1
Just enable Google Places API for Android in your Google Developer Console.
Don't forget to specify your API key at AndroidManifest
:
<meta-data
android:name="com.google.android.geo.API_KEY"
android:value="ADD_YOUR_API_KEY_HERE" />
Solution 2
I believe that when Google made changes to their API's on 04/20/2015 they modified the places API that the PlacePicker inherently uses. The solution that worked for me is as follows:
- Go to your Google Developer Console (https://console.developers.google.com/project)
- Navigate to your project if you have made one. If not go here: https://developers.google.com/console/help/
- Navigate to "APIs & auth" then to "Credentials" on the left side of the console. I don't have enough rep to post a picture for guidance...
- Create a new Key, this appears under Public API access in your Credentials window. You will need your SHA1 Fingerprint to receive the key. Directions that I used are here: How to get the SHA-1 fingerprint certificate in Android Studio for debug mode?
- The location that you input your SHA1 Fingerprint should be in this format: (Your Key, 20 Hex values separated by ":");com.yourpackage.yourapp
- Copy your API Key that is generated. You will need to add the fingerprint of each developer in the same format above for it to work for other developers.
- Add your key to your AndroidManifest.xml
<application ...
<meta-data
android:name="com.google.android.geo.API_KEY"
android:value="@string/google_api_key" />
... </application>
<string name="google_api_key">yourkeyhere</string>
- Clean and Rebuild your project. Should be working now. I don't think I left any steps out... Please comment if I have.
Solution 3
I have the same issue. I add Google map key
<meta-data
android:name="com.google.android.maps.v2.API_KEY"
android:value="@string/google_maps_key"/>` in manifest file.
The Place Picker closes immediately. Then, i also add
<meta-data
android:name="com.google.android.geo.API_KEY"
android:value="@string/google_api_key" />
The Place Picker shows, but when i open map, my app was force close, and log like below.
RuntimeException: The API key can only be specified once. It is recommended that you use the meta-data tag with the name: com.google.android.maps.v2.API_KEY in the <application> element of AndroidManifest.xml
at com.google.maps.api.android.lib6.d.fb.a(Unknown Source)
at com.google.maps.api.android.lib6.a.g.a(Unknown Source)
at com.google.android.gms.maps.internal.CreatorImpl.b(Unknown Source)
at com.google.android.gms.maps.internal.CreatorImpl.b(Unknown Source)
at com.google.android.gms.maps.internal.i.onTransact(SourceFile:62)
at android.os.Binder.transact(Binder.java:364)
at com.google.android.gms.maps.internal.zzc$zza$zza.zzj(Unknown Source)
at com.google.android.gms.maps.SupportMapFragment$zzb.zzqs(Unknown Source)
at com.google.android.gms.maps.SupportMapFragment$zzb.zza(Unknown Source)
at com.google.android.gms.dynamic.zza.zza(Unknown Source)
at com.google.android.gms.dynamic.zza.onCreate(Unknown Source)
at com.google.android.gms.maps.SupportMapFragment.onCreate(Unknown Source)
at android.support.v4.app.Fragment.performCreate(Fragment.java:1763)
at android.support.v4.app.FragmentManagerImpl.moveToState(FragmentManager.java:915)
at android.support.v4.app.FragmentManagerImpl.moveToState(FragmentManager.java:1136)
at android.support.v4.app.BackStackRecord.run(BackStackRecord.java:739)
at android.support.v4.app.FragmentManagerImpl.execPendingActions(FragmentManager.java:1499)
at android.support.v4.app.FragmentManagerImpl$1.run(FragmentManager.java:456)
at android.os.Handler.handleCallback(Handler.java:733)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:136)
at android.app.ActivityThread.main(ActivityThread.java:5103)
at java.lang.reflect.Method.invokeNative(Native Method)
at java.lang.reflect.Method.invoke(Method.java:515)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:790)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:606)
at dalvik.system.NativeStart.main(Native Method)
I try to remove
<meta-data
android:name="com.google.android.maps.v2.API_KEY"
android:value="@string/google_maps_key"/>`
but keep
<meta-data
android:name="com.google.android.geo.API_KEY"
android:value="@string/google_api_key" />
And It's working well, now, and I don't know why.
Solution 4
replace your meta data tag in the mainfeast .. it works for me ..
<meta-data
android:name="com.google.android.maps.v2.API_KEY"
android:value="YOUR ANDROID KEY" />
with
<meta-data
android:name="com.google.android.geo.API_KEY"
android:value="YOUR ANDROID KEY" />
good luck
Solution 5
I had the same problems using the Places API. At the end, I figured out that there are different Google Places APIs especially for Android. Consequently, the API-Key that I was using was simply for the non-Android version.
Generate your key using this link: https://developers.google.com/places/android-api/signup
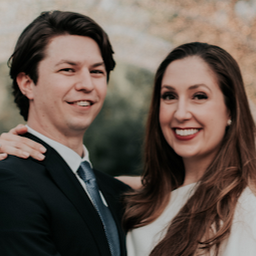
Cole Laidlaw
Updated on June 23, 2022Comments
-
Cole Laidlaw almost 2 years
I am developing an Android Application and was looking into Google Places for some functionality within the application. Google has recently released the PlacePicker feature which is what I am using. I have followed the Google guidelines and quick start guides to the "T". The functionality was working amazing as of three hours ago and now when I go to test it, it launches and then immediately closes with no errors thrown in the stack trace. Here is how I am using the code:
This is within a fragment.
// The Location Selection from Google Place Picker where.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { try { launchPlace(); } catch (GooglePlayServicesNotAvailableException | GooglePlayServicesRepairableException e) { e.printStackTrace(); } } });
This is within the fragments onCreateView.
The method launchPlace() is defined as follows.
// Start Google Place Picker Code ************************************************************** public void launchPlace() throws GooglePlayServicesNotAvailableException, GooglePlayServicesRepairableException { PLACE_PICKER_REQUEST = 1; PlacePicker.IntentBuilder builder = new PlacePicker.IntentBuilder(); Context context = getActivity().getApplicationContext(); startActivityForResult(builder.build(context), PLACE_PICKER_REQUEST); } public void onActivityResult(int requestCode, int resultCode, Intent data) { String badLocation = "That location is not valid for this app, please select a valid location"; if (requestCode == PLACE_PICKER_REQUEST) { if (resultCode == Activity.RESULT_OK) { place = PlacePicker.getPlace(data, getActivity().getApplicationContext()); if (place.getPlaceTypes().contains(34)) { if (place.getPlaceTypes().contains(9) || place.getPlaceTypes().contains(15) || place.getPlaceTypes().contains(38) || place.getPlaceTypes().contains(67) || place.getPlaceTypes().contains(79)) { where.setText(place.getName()); loc = true; } else { Toast.makeText(getActivity().getApplicationContext(), badLocation, Toast.LENGTH_LONG).show(); try { launchPlace(); } catch (GooglePlayServicesNotAvailableException | GooglePlayServicesRepairableException e) { e.printStackTrace(); } } } } } }
This code worked previously (3 hours ago) and now does not. Here is what LogCat says: (Android Studio)
04-21 15:48:02.320 5045-5045/com.siliconmindtech.trofi D/ViewRootImpl﹕ ViewPostImeInputStage ACTION_DOWN 04-21 15:48:02.680 5045-5124/com.siliconmindtech.trofi D/OpenGLRenderer﹕ endAllStagingAnimators on 0xa1a55600 (InsetDrawable) with handle 0xa185dff0 04-21 15:48:05.000 5045-5121/com.siliconmindtech.trofi I/System.out﹕ (HTTPLog)-Static: isSBSettingEnabled false 04-21 15:48:05.060 5045-5045/com.siliconmindtech.trofi I/Timeline﹕ Timeline: Activity_idle id: android.os.BinderProxy@215c768a time:9757022
If anyone knows of a way to help with this that would be amazing, I know that Google PlacePicker is quite new but I am a novice Android Developer and feel like someone has a better understanding than I. Any and all help would be appreciated. If more information is required please let me know. We use GitHub so I have reverted all the way back to when it once worked, yet nothing changed, it still closes (the picker, not the app). I have enabled the Google Places API and Google Places API for Android in the developer console, no help there either. It gets to the "Updating your location" screen then stops.
EDIT: This is the entire LogCat, from launch (On a Samsung Galaxy S5) to the PlacePicker starting (One navigation prior to that action) then closing and returning to the app. Is this what you meant?
04-21 16:56:26.740 27318-27318/com.siliconmindtech.trofi D/ResourcesManager﹕ creating new AssetManager and set to /data/app/com.siliconmindtech.trofi-1/base.apk 04-21 16:56:26.910 27318-27318/com.siliconmindtech.trofi D/AbsListView﹕ Get MotionRecognitionManager 04-21 16:56:26.910 27318-27340/com.siliconmindtech.trofi I/System.out﹕ (HTTPLog)-Static: isSBSettingEnabled false 04-21 16:56:26.910 27318-27340/com.siliconmindtech.trofi I/System.out﹕ (HTTPLog)-Static: isShipBuild true 04-21 16:56:26.910 27318-27340/com.siliconmindtech.trofi I/System.out﹕ (HTTPLog)-Thread-13137-1066627414: SmartBonding Enabling is false, SHIP_BUILD is true, log to file is false, DBG is false 04-21 16:56:26.910 27318-27340/com.siliconmindtech.trofi I/System.out﹕ (HTTPLog)-Static: isSBSettingEnabled false 04-21 16:56:26.910 27318-27318/com.siliconmindtech.trofi D/AbsListView﹕ Get MotionRecognitionManager 04-21 16:56:26.940 27318-27318/com.siliconmindtech.trofi D/Activity﹕ performCreate Call secproduct feature valuefalse 04-21 16:56:26.940 27318-27318/com.siliconmindtech.trofi D/Activity﹕ performCreate Call debug elastic valuetrue 04-21 16:56:26.950 27318-27318/com.siliconmindtech.trofi D/AbsListView﹕ Get MotionRecognitionManager 04-21 16:56:26.970 27318-27350/com.siliconmindtech.trofi D/OpenGLRenderer﹕ Render dirty regions requested: true 04-21 16:56:27.030 27318-27350/com.siliconmindtech.trofi I/Adreno-EGL﹕ <qeglDrvAPI_eglInitialize:410>: EGL 1.4 QUALCOMM build: () OpenGL ES Shader Compiler Version: E031.25.01.03 Build Date: 10/28/14 Tue Local Branch: LA.BF.1.1_RB1_20141028_021_patches2 Remote Branch: Local Patches: Reconstruct Branch: 04-21 16:56:27.030 27318-27350/com.siliconmindtech.trofi I/OpenGLRenderer﹕ Initialized EGL, version 1.4 04-21 16:56:27.050 27318-27350/com.siliconmindtech.trofi I/OpenGLRenderer﹕ HWUI protection enabled for context , &this =0xa23090d8 ,&mEglDisplay = 1 , &mEglConfig = 8 04-21 16:56:27.050 27318-27350/com.siliconmindtech.trofi D/OpenGLRenderer﹕ Enabling debug mode 0 04-21 16:56:27.050 27318-27340/com.siliconmindtech.trofi I/System.out﹕ KnoxVpnUidStorageknoxVpnSupported API value returned is false 04-21 16:56:27.240 27318-27350/com.siliconmindtech.trofi V/RenderScript﹕ Application requested CPU execution 04-21 16:56:27.260 27318-27350/com.siliconmindtech.trofi V/RenderScript﹕ 0xa2377a00 Launching thread(s), CPUs 4 04-21 16:56:27.310 27318-27318/com.siliconmindtech.trofi I/Timeline﹕ Timeline: Activity_idle id: android.os.BinderProxy@344b9848 time:13859270 04-21 16:56:29.700 27318-27318/com.siliconmindtech.trofi D/ViewRootImpl﹕ ViewPostImeInputStage ACTION_DOWN 04-21 16:56:31.850 27318-27318/com.siliconmindtech.trofi D/ViewRootImpl﹕ ViewPostImeInputStage ACTION_DOWN 04-21 16:56:31.970 27318-27318/com.siliconmindtech.trofi I/Places﹕ Got here! 04-21 16:56:32.300 27318-27350/com.siliconmindtech.trofi D/OpenGLRenderer﹕ endAllStagingAnimators on 0xa196d600 (InsetDrawable) with handle 0xaf7fc350 04-21 16:56:32.740 27318-27318/com.siliconmindtech.trofi I/Timeline﹕ Timeline: Activity_idle id: android.os.BinderProxy@344b9848 time:13864703
The above LogCat is Verbose.
Solution: Don't be a newbie like me, I had forgotten to add my Google API Key to my android_manifest.xml... I apologize.