Greatest GCD between some numbers
Solution 1
There is a solution that would take O(n):
Let our numbers be a_i
. First, calculate m=a_0*a_1*a_2*...
. For each number a_i, calculate gcd(m/a_i, a_i)
. The number you are looking for is the maximum of these values.
I haven't proved that this is always true, but in your example, it works:
m=2*4*5*15=600,
max(gcd(m/2,2), gcd(m/4,4), gcd(m/5,5), gcd(m/15,15))=max(2, 2, 5, 5)=5
NOTE: This is not correct. If the number a_i
has a factor p_j
repeated twice, and if two other numbers also contain this factor, p_j
, then you get the incorrect result p_j^2
insted of p_j
. For example, for the set 3, 5, 15, 25
, you get 25
as the answer instead of 5
.
However, you can still use this to quickly filter out numbers. For example, in the above case, once you determine the 25, you can first do the exhaustive search for a_3=25
with gcd(a_3, a_i)
to find the real maximum, 5
, then filter out gcd(m/a_i, a_i), i!=3
which are less than or equal to 5
(in the example above, this filters out all others).
Added for clarification and justification:
To see why this should work, note that gcd(a_i, a_j)
divides gcd(m/a_i, a_i)
for all j!=i
.
Let's call gcd(m/a_i, a_i)
as g_i
, and max(gcd(a_i, a_j),j=1..n, j!=i)
as r_i
. What I say above is g_i=x_i*r_i
, and x_i
is an integer. It is obvious that r_i <= g_i
, so in n
gcd operations, we get an upper bound for r_i
for all i
.
The above claim is not very obvious. Let's examine it a bit deeper to see why it is true: the gcd of a_i
and a_j
is the product of all prime factors that appear in both a_i
and a_j
(by definition). Now, multiply a_j
with another number, b
. The gcd of a_i
and b*a_j
is either equal to gcd(a_i, a_j)
, or is a multiple of it, because b*a_j
contains all prime factors of a_j
, and some more prime factors contributed by b
, which may also be included in the factorization of a_i
. In fact, gcd(a_i, b*a_j)=gcd(a_i/gcd(a_i, a_j), b)*gcd(a_i, a_j)
, I think. But I can't see a way to make use of this. :)
Anyhow, in our construction, m/a_i
is simply a shortcut to calculate the product of all a_j
, where j=1..1, j!=i
. As a result, gcd(m/a_i, a_i)
contains all gcd(a_i, a_j)
as a factor. So, obviously, the maximum of these individual gcd results will divide g_i
.
Now, the largest g_i
is of particular interest to us: it is either the maximum gcd itself (if x_i
is 1), or a good candidate for being one. To do that, we do another n-1
gcd operations, and calculate r_i
explicitly. Then, we drop all g_j
less than or equal to r_i
as candidates. If we don't have any other candidate left, we are done. If not, we pick up the next largest g_k
, and calculate r_k
. If r_k <= r_i
, we drop g_k
, and repeat with another g_k'
. If r_k > r_i
, we filter out remaining g_j <= r_k
, and repeat.
I think it is possible to construct a number set that will make this algorithm run in O(n^2) (if we fail to filter out anything), but on random number sets, I think it will quickly get rid of large chunks of candidates.
Solution 2
You can use the Euclidean Algorithm to find the GCD of two numbers.
while (b != 0)
{
int m = a % b;
a = b;
b = m;
}
return a;
Solution 3
If you want an alternative to the obvious algorithm, then assuming your numbers are in a bounded range, and you have plenty of memory, you can beat O(N^2) time, N being the number of values:
- Create an array of a small integer type, indexes 1 to the max input. O(1)
- For each value, increment the count of every element of the index which is a factor of the number (make sure you don't wraparound). O(N).
- Starting at the end of the array, scan back until you find a value >= 2. O(1)
That tells you the max gcd, but doesn't tell you which pair produced it. For your example input, the computed array looks like this:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
4 2 1 1 2 0 0 0 0 0 0 0 0 0 1
I don't know whether this is actually any faster for the inputs you have to handle. The constant factors involved are large: the bound on your values and the time to factorise a value within that bound.
You don't have to factorise each value - you could use memoisation and/or a pregenerated list of primes. Which gives me the idea that if you are memoising the factorisation, you don't need the array:
- Create an empty set of int, and a best-so-far value 1.
- For each input integer:
- if it's less than or equal to best-so-far, continue.
- check whether it's in the set. If so, best-so-far = max(best-so-far, this-value), continue. If not:
- add it to the set
- repeat for all of its factors (larger than best-so-far).
Add/lookup in a set could be O(log N), although it depends what data structure you use. Each value has O(f(k)) factors, where k is the max value and I can't remember what the function f is...
The reason that you're finished with a value as soon as you encounter it in the set is that you've found a number which is a common factor of two input values. If you keep factorising, you'll only find smaller such numbers, which are not interesting.
I'm not quite sure what the best way is to repeat for the larger factors. I think in practice you might have to strike a balance: you don't want to do them quite in decreasing order because it's awkward to generate ordered factors, but you also don't want to actually find all the factors.
Even in the realms of O(N^2), you might be able to beat the use of the Euclidean algorithm:
Fully factorise each number, storing it as a sequence of exponents of primes (so for example 2 is {1}, 4 is {2}, 5 is {0, 0, 1}, 15 is {0, 1, 1}). Then you can calculate gcd(a,b) by taking the min value at each index and multiplying them back out. No idea whether this is faster than Euclid on average, but it might be. Obviously it uses a load more memory.
Solution 4
The optimisations I can think of is
1) start with the two biggest numbers since they are likely to have most prime factors and thus likely to have the most shared prime factors (and thus the highest GCD).
2) When calculating the GCDs of other pairs you can stop your Euclidean algorithm loop if you get below your current greatest GCD.
Off the top of my head I can't think of a way that you can work out the greatest GCD of a pair without trying to work out each pair individually (and optimise a bit as above).
Disclaimer: I've never looked at this problem before and the above is off the top of my head. There may be better ways and I may be wrong. I'm happy to discuss my thoughts in more length if anybody wants. :)
Solution 5
There is no O(n log n)
solution to this problem in general. In fact, the worst case is O(n^2)
in the number of items in the list. Consider the following set of numbers:
2^20 3^13 5^9 7^2*11^4 7^4*11^3
Only the GCD of the last two is greater than 1, but the only way to know that from looking at the GCDs is to try out every pair and notice that one of them is greater than 1.
So you're stuck with the boring brute-force try-every-pair approach, perhaps with a couple of clever optimizations to avoid doing needless work when you've already found a large GCD (while making sure that you don't miss anything).
Related videos on Youtube
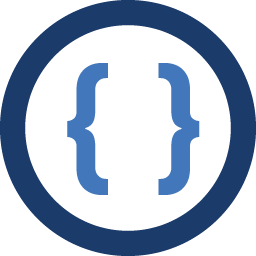
Admin
Updated on May 02, 2022Comments
-
Admin about 2 years
We've got some nonnegative numbers. We want to find the pair with maximum gcd. actually this maximum is more important than the pair! For example if we have:
2 4 5 15
gcd(2,4)=2
gcd(2,5)=1
gcd(2,15)=1
gcd(4,5)=1
gcd(4,15)=1
gcd(5,15)=5
The answer is 5.
-
Admin over 13 yearsI know this. but what about more than two? I want the maximum gcd between a pair of these numbers. for example if numbers are:2,4,5,10 the answer is 5, but of course it's not efficient to calculate gcd for each pair
-
Wildcat over 13 yearsFor more than two you can use recursive formula gcd(a[1], ..., a[n]) = gcd(gcd(a[1], ..., a[n - 1]), a[n]).
-
Admin over 13 yearsThanks! These were really really helpful.
-
anshul garg almost 7 yearsThis is n*n algorithm
-
Mircea almost 7 yearsThanks! Indeed, still in N^2.