gulp sass source map
13,862
Solution 1
Try this code for gulp task 'sass':
gulp.task('sass', function() {
return gulp.src('scss/**/*.scss')
.pipe(sourcemaps.init())
.pipe(sass())
.pipe(sourcemaps.write('.'))
.pipe(gulp.dest('assets/css'))
.pipe(bs.reload({
stream: true
}));
});
First init sourcemaps then compile sass() after that write sourcemap in the same folder ('.')
Regards
Solution 2
I'm using this task since 5 months everyday and works fine,
const gulp = require('gulp'),
autoprefixer = require('gulp-autoprefixer'),
plumber = require('gulp-plumber'),
sass = require('gulp-sass'),
sourcemaps = require('gulp-sourcemaps');
var sassSourcePath = 'YourPath/scss/**/*.scss',
cssDestPath = 'YourPath/css/';
gulp.task('sass', () => {
return gulp
.src(sassSourcePath)
.pipe(plumber())
.pipe(sourcemaps.init())
.pipe(sass({outputStyle: 'compressed'}).on('error', sass.logError))
.pipe(sourcemaps.write({includeContent: false}))
.pipe(sourcemaps.init({loadMaps: true}))
.pipe(autoprefixer({ browser: ['last 2 version', '> 5%'] }))
.pipe(sourcemaps.write('.'))
.pipe(gulp.dest(cssDestPath));
});
Also recommend you the require('gulp-csso')
for the production version
Solution 3
A complete solution for gulp-sass, map, count all files, minify:
./sass/partial_folders/index.scss
@import 'base/_reset';
@import 'helpers/_variables';
@import 'helpers/_mixins';
@import 'helpers/_functions';
@import 'base/_typography';
etc..
./gulpfile.js
var gulp = require('gulp');
var sass = require('gulp-sass');
var concat = require('gulp-concat');
var uglifycss = require('gulp-uglifycss');
var sourcemaps = require('gulp-sourcemaps');
gulp.task('styles', function(){
return gulp
.src('sass/**/*.scss')
.pipe(sourcemaps.init())
.pipe(sass().on('error', sass.logError))
.pipe(concat('styles.css'))
.pipe(uglifycss({
"maxLineLen": 80,
"uglyComments": true
}))
.pipe(sourcemaps.write('.'))
.pipe(gulp.dest('./build/css/'));
});
gulp.task('default', function(){
gulp.watch('sass/**/*.scss', ['styles']);
})
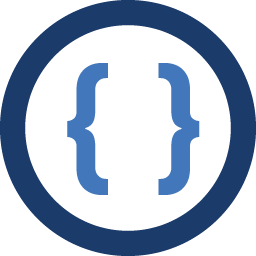
Author by
Admin
Updated on June 11, 2022Comments
-
Admin almost 2 years
I need help adding source map to SASS compiler in the same CSS output folder. Till now, I got to install gulp-sourcemaps module within
gulpfile.js
but couldn't know success to bindsourcemaps.write
as gulp.task.Any help is much appreciated :)
var gulp = require('gulp'); var sass = require('gulp-sass'); var sourcemaps = require('gulp-sourcemaps'); var bs = require('browser-sync').create(); gulp.task('browser-sync', ['sass'], function() { bs.init({ server: { baseDir: "./" }, proxy: { target: "localhost:8080", // can be [virtual host, sub-directory, localhost with port] ws: true // enables websockets } }); }); gulp.task('sass', function() { return gulp.src('scss/**/*.scss') .pipe(sourcemaps.init()) .pipe(sass()) .pipe(sourcemaps.write('.')) .pipe(gulp.dest('assets/css')) .pipe(bs.reload({ stream: true })); }); gulp.task('watch', ['browser-sync'], function () { gulp.watch("scss/*.scss", ['sass']); gulp.watch("*.php").on('change', bs.reload); });