Handling api calls in Redux with Axios
Solution 1
axios
is the promise so you need to use then
to get your result. You should request your api in a separate file and call your action when the result comes back.
//WebAPIUtil.js
axios.get('http://localhost:3000/flug')
.then(function(result){
YourAction.getAllFlights(result)
});
In your action file will be like this :
export function getAllFlights(request) {
console.log(request);
return {
type: FETCH_FLIGHT,
payload: request
};
}
Solution 2
You can do this with thunk. https://github.com/gaearon/redux-thunk
You can dispatch an action in your then
and it will update state when it gets a response from the axios call.
export function someFunction() {
return(dispatch) => {
axios.get(URL)
.then((response) => {dispatch(YourAction(response));})
.catch((response) => {return Promise.reject(response);});
};
}
Solution 3
I also think the best way to do this is by redux-axios-middleware. The setup can be a bit tricky as your store should be configured in a similar way:
import { createStore, applyMiddleware } from 'redux';
import axiosMiddleware from 'redux-axios-middleware';
import axios from 'axios';
import rootReducer from '../reducers';
const configureStore = () => {
return createStore(
rootReducer,
applyMiddleware(axiosMiddleware(axios))
);
}
const store = configureStore();
And your action creators now look like this:
import './axios' // that's your axios.js file, not the library
export const FETCH_FLIGHT = 'FETCH_FLIGHT';
export const getAllFlights = () => {
return {
type: FETCH_FLIGHT,
payload: {
request: {
method: 'post', // or get
url:'http://localhost:3000/flug'
}
}
}
}
Solution 4
The best way to solve this is by adding redux middlewares http://redux.js.org/docs/advanced/Middleware.html for handling all api requests.
https://github.com/svrcekmichal/redux-axios-middleware is a plug and play middleware you can make use of.
Solution 5
I took care of this task like so:
import axios from 'axios';
export const receiveTreeData = data => ({
type: 'RECEIVE_TREE_DATA', data,
})
export const treeRequestFailed = (err) => ({
type: 'TREE_DATA_REQUEST_FAILED', err,
})
export const fetchTreeData = () => {
return dispatch => {
axios.get(config.endpoint + 'tree')
.then(res => dispatch(receiveTreeData(res.data)))
.catch(err => dispatch(treeRequestFailed(err)))
}
}
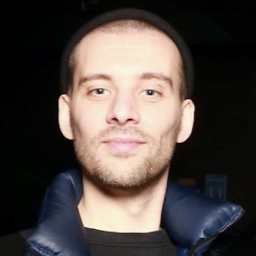
Steinar
Updated on March 16, 2020Comments
-
Steinar about 4 years
Good evening everybody!
I'm a total beginner in React and Redux so please bear with me if this sounds totally stupid. I'm trying to learn how I can perform some API calls in Redux and it's not going all to well. When I console log the request from the action creator the promise value is always "undefined" so I'm not sure if I'm doing this correctly.
My goal is to grab the information from the data inside the payload object and display them inside the component. I've been trying to get this to work for the past days and I'm totally lost.
I'm using Axios for and redux-promise to handle the call.
Any help will be greatly appreciated.
Here's the output from the console.
Action Creator
import axios from 'axios'; export const FETCH_FLIGHT = 'FETCH_FLIGHT'; export function getAllFlights() { const request = axios.get('http://localhost:3000/flug'); console.log(request); return { type: FETCH_FLIGHT, payload: request }; }
Reducer
import { FETCH_FLIGHT } from '../actions/index'; export default function(state = [], action) { switch (action.type) { case FETCH_FLIGHT: console.log(action) return [ action.payload.data, ...state ] } return state; }
Component
import React from 'react'; import { Component } from 'react'; import { connect } from 'react-redux'; import { bindActionCreators } from 'redux'; import { getAllFlights } from '../actions/index'; import Destinations from './Destinations'; class App extends Component { componentWillMount(){ this.props.selectFlight(); } constructor(props) { super(props); this.state = { }; } render() { return ( <div> </div> ); } function mapStateToProps(state) { return { dest: state.icelandair }; } function mapDispatchToProps(dispatch) { return bindActionCreators({ selectFlight: getAllFlights }, dispatch); } export default connect(mapStateToProps, mapDispatchToProps)(App);