Haskell Creating list of numbers
12,832
Solution 1
Actually, I am not sure if I get your idea. But Is this what you want?
generator list = list ++ generator next
where
next = (map (\n -> 2 * n + 1) list) ++ (map (\n -> 3 * n + 1) list)
Oh, you can use generator [1] to fire up. like this:
take 100 $ generator [1]
Solution 2
merge xs [] = xs
merge [] ys = ys
merge (x:xs) (y:ys) | x == y = x : merge xs ys
| x < y = x : merge xs (y:ys)
| otherwise = y : merge (x:xs) ys
print $ take 10 $ merge [1,3..] [1,4..]
--[1,3,4,5,7,9,10,11,13,15]
Solution 3
As luqui said, we could use info such as do duplicates matter and does order matter. If the answers are no and no then a simple concatMap works fine:
myList = 1 : concatMap (\n -> 2*n+1 : 3*n+1 : []) myList
Results in:
> take 20 myList
[1,3,4,7,10,9,13,15,22,21,31,19,28,27,40,31,46,45,67,43]
If the answers are yes and yes then I imagine it could be cleaner, but this is sufficient:
myList = abs
where
abs = merge as bs
as = 1 : map (\n -> 2*n+1) abs
bs = 1 : map (\n -> 3*n+1) abs
merge (x:xs) (y:ys)
| x == y = x : merge xs ys
| x < y = x : merge xs (y:ys)
| otherwise = y : merge (x:xs) ys
Results in:
> take 20 myList
[1,3,4,7,9,10,13,15,19,21,22,27,28,31,39,40,43,45,46,55]
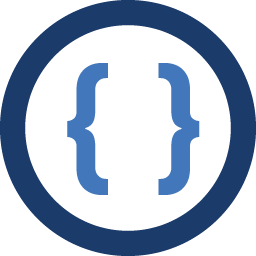
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
Hi Im new to Haskell and wish to write a simple code. I want to write a function which creates a list of numbers. Where it starts of with 1 and increase with 2n+1 and 3n+1 so for example output should be like take 6 myList = [1,3,4,7,9,10]
I think i need to use recursion but not sure how to do it in list format.
Any help will be appreciated. Thanks