head node in linked list in c
Solution 1
Malloc is only required when dynamically allocating memory. If you write something like struct node head
inside of a method, the head node will be allocated on the stack, and will live (and die) there. Thus, you could write data to this node, but it will die once the method finishes.
Or, you could define the head globally, in which case it will be statically allocated and will live forever until the program exits. Malloc is only required when you want to create a piece of memory which will live on after the function has exited. It is only cleared when you call free
on the pointer.
Solution 2
You are right, If you haven't initialize the head, then head will not point to next node so you have to initialize it that is why second implementation is used unanimously. If you are using first method, still you need to initialize it and if you are initializing it why now use its data variable too.
Solution 3
You are missing a C dynamic memory allocation:
struct node *head = malloc(sizeof (struct node));
if (!head) { perror("malloc node"); exit(EXIT_FAILURE); };
head->next = NULL;
// initialize other fields of head
Don't forget to initialize all the fields of head
! Read malloc(3)
You could have a global variable full_list
containing the first pointer, e.g.
if (!full_list)
full_list = head;
See also this... Read linked list wikipage.
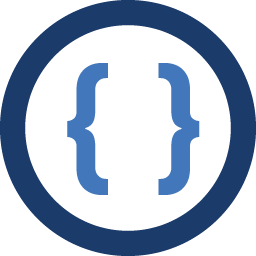
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
while studying linked list in c,i came across 2 different implementations of the head node. say if the following is the structure used:
struct node { int data; struct node *next; }
then the first implementation is where the head node is just a dummy node with no actual data in it, but just a link to another node(the first actual node with data) like this:
struct node *head; head->next = NULL; //head->next would then be linked to the first node.
the second implementaton is the one where the head node is the first actual node with data in it which is stored using malloc command to allocate space to it.
my question is, how can we use the "head->next" in the first implementation where we havent allocated space for head using malloc at all? because as far as i know(correct me if am wrong), the two fields of the node can only be used once space has been allocated to that node.