HEAD with WebClient?
10,208
Solution 1
You are right WebClient does not support this. You can use HttpWebRequest and set the method to HEAD if you want this functionality:
System.Net.WebRequest request = System.Net.WebRequest.Create(uri);
request.Method = "HEAD";
request.GetResponse();
Solution 2
Another way is to inherit from WebClient and override GetWebRequest(Uri address).
public class ExWebClient : WebClient
{
public string Method
{
get;
set;
}
protected override WebRequest GetWebRequest(Uri address)
{
WebRequest webRequest = base.GetWebRequest(address);
if (!string.IsNullOrEmpty(Method))
webRequest.Method = Method;
return webRequest;
}
}
Solution 3
Most web servers that I request from will accept this method. Not every web server does though. IIS6, for example, will honor the request method SOMETIMES.
This is the status code that is returned when a method isn't allowed...
catch (WebException webException)
{
if (webException.Response != null)
{
//some webservers don't allow the HEAD method...
if (((HttpWebResponse) webException.Response).StatusCode == HttpStatusCode.MethodNotAllowed)
Thanks, Mike
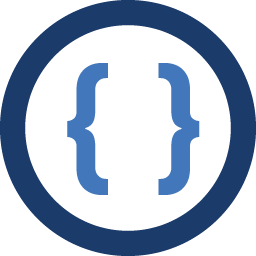
Author by
Admin
Updated on June 20, 2022Comments
-
Admin almost 2 years
I am going to assume the answer is no but.... Is there a way to use WebClient to send the HEAD method and return the headers as a string or something similar?