Hero animation and scroll to position between two list in Flutter
517
this is what i've done, try:
import 'package:flutter/material.dart';
import 'package:scrollable_positioned_list/scrollable_positioned_list.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
@override
_HomePageState createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
@override
void initState() {
super.initState();
}
@override
void dispose() {
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: GridView.count(
crossAxisCount: 3,
children: List.generate(99, (index) {
return Container(
width: double.infinity,
height: double.infinity,
child: GestureDetector(
onTap: () {
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => ListPage(index: index)),
);
},
child: Hero(
tag: 'photo$index',
child: Image.network(
'https://images.unsplash.com/photo-1628701621033-50564c683bb0?ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&ixlib=rb-1.2.1&auto=format&fit=crop&w=800&q=80',
fit: BoxFit.cover,
),
),
),
);
}),
),
);
}
}
class ListPage extends StatefulWidget {
final int index;
ListPage({Key key, @required this.index}) : super(key: key);
@override
_ListPageState createState() => _ListPageState();
}
class _ListPageState extends State<ListPage> {
final ItemScrollController itemScrollController = ItemScrollController();
final ItemPositionsListener itemPositionsListener =
ItemPositionsListener.create();
@override
void initState() {
super.initState();
}
@override
void dispose() {
super.dispose();
}
@override
Widget build(BuildContext context) {
return ScrollablePositionedList.builder(
initialScrollIndex: widget.index,
itemCount: 99,
itemBuilder: (context, index) {
return Column(
children: [
AspectRatio(
aspectRatio: 1,
child: Hero(
tag: 'photo$index',
child: Image.network(
'https://images.unsplash.com/photo-1628701621033-50564c683bb0?ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&ixlib=rb-1.2.1&auto=format&fit=crop&w=800&q=80',
fit: BoxFit.cover,
),
),
),
Container(
height: 50,
width: MediaQuery.of(context).size.width,
child: Text('$index'),
),
],
);
},
itemScrollController: itemScrollController,
itemPositionsListener: itemPositionsListener,
);
}
}
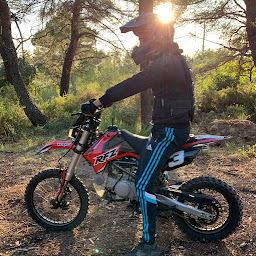
Author by
Tom3652
Updated on December 31, 2022Comments
-
Tom3652 over 1 year
I am displaying files as thumbnails such as the Instagram user's profile.
When i click on an item (thumbnail) i have to perform a
Hero
animation that will reveal the full screen page.I also want to open the full screen page with the whole list which is directly starting at the given index of the selected post, exactly like in Instagram.
Here is a short GIF that shows better what i mean :
I have tried the scroll_to_index package but this does not meet my needs (animation is broken and too slow).
How can i achieve this please ?
-
Tom3652 over 2 yearsThanks for your answer, but the package you are using is not null safety. I have not specified it in my question my bad. I would like the logic to work with any scrollable list actually but if you have a working null safety example i will try it right away !
-
Jim over 2 yearsit did have null safety version, pub.dev/packages/scrollable_positioned_list/versions/…
-
Tom3652 over 2 yearsIt's working very fine thank you. I had a conflict with
SmartRefresher
package in case others faces weird scrolling issues withScrollablePositionedList
(it was scrolling to bottom despite the initial index, that's why i took me so long to validate. -
Jim over 2 yearsglad that helps, TGIF!