Hibernate naming strategy changing table names
Solution 1
spring.jpa.hibernate.naming.physical-strategy=org.hibernate.boot.model.naming.PhysicalNamingStrategyStandardImpl
it worked for me. here are the versions i'm using:
Spring Boot (v1.4.2.RELEASE)
Hibernate Core {5.0.11.Final}
Solution 2
For one who wants to upper case in Postgresql and Spring boot 1.5.2
public class CustomDatabaseIdentifierNamingStrategy extends org.springframework.boot.orm.jpa.hibernate.SpringPhysicalNamingStrategy implements Serializable {
public static final long serialVersionUID = 1L;
public static final CustomDatabaseIdentifierNamingStrategy INSTANCE = new CustomDatabaseIdentifierNamingStrategy();
@Override
public Identifier toPhysicalTableName(Identifier name, JdbcEnvironment context) {
return new Identifier(name.getText().toUpperCase(), true);
}
@Override
public Identifier toPhysicalColumnName(Identifier name, JdbcEnvironment context) {
return new Identifier(name.getText().toUpperCase(), true);
}
}
Solution 3
If you are providing @Table and @Column annotation in your entity classes with names provided with an underscore i.e. user_id i.e. @Column(name="user_id"), it will take the column name as user_id; if you give it as userid then it will change to user_id if you use no strategy or implicit strategy (specifically spring.jpa.hibernate.naming.implicit-strategy=org.hibernate.boot.model.naming.ImplicitNamingStrategyLegacyHbmImpl). So, if you want a strategy where the entity attribute name changes to one with underscore and lowercase letters i.e. something from userId to user_id, you should use implicit or no strategy (which actually uses implicit strategy).
If you don't want your naming strategy to add an underscore to the column name or class name, then the strategy that you need to use would look like: spring.jpa.hibernate.naming.physical-strategy=org.hibernate.boot.model.naming.PhysicalNamingStrategyStandardImpl. The things that you provide in annotations @Table and @Column’s name attribute would remain as it is.
If you don't want to provide annotations and want to manually handle the table name and column names, you should extend the class org.hibernate.boot.model.naming.PhysicalNamingStrategyStandardImpl and override the required methods. If you still use annotations for some of the cases here, remember the overridden methods will apply on the names written in those annotations. spring.jpa.hibernate.naming.physical-strategy=example.CustomStrategy
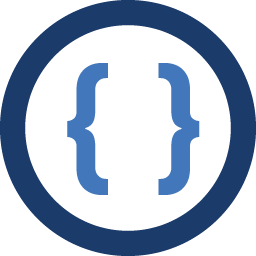
Admin
Updated on July 30, 2022Comments
-
Admin almost 2 years
I'm a little bit confused by hibernates (version 5.1) naming strategy - namely it changes my table name and I'd like to avoid that. Also -
spring.jpa.hibernate.naming_strategy
seems to be deprecated according to intelij, but I can't find a (nother) way of configuring it correctly.I have the following configuration in application.properties:
spring.jpa.hibernate.naming_strategy=org.hibernate.cfg.EJB3NamingStrategy spring.jpa.properties.hibernate.dialect = org.hibernate.dialect.MySQL5Dialect spring.jpa.properties.hibernate.enable_lazy_load_no_trans=true spring.jpa.properties.hibernate.current_session_context_class=thread
The first one is marked as depracted, as said.
Now I have an entity:
@Entity @Table(name = "usaUploadTable", schema = "usertable201", catalog = "") public class UsaUploadTable { .... }
The table name is, like in
@Table(name = "")
usaUploadTable.Now when I run my application, I get
Table 'usertable201.usa_upload_table' doesn't exist
which is correct - it isn't named like how hibernate is changing it.
What can I do to make hibernate use my table name correctly?
Edit:
I've also tried
DefaultNamingStrategy ImprovedNamingStrategy
All of them change it
Versions:
spring-boot-1.4.0.RELEASE hibernate 5.1 javax-transaction-api 1.2 hibernate-validator 5.2.4 javassist 3.20
-
Roland Illig over 6 yearsBe careful when running the above code on a Turkish server.
toUpperCase()
will convertitems
toİTEMS
(latin capital letter i with dot above). To fix this, always usetoUpperCase(Locale.ROOT)
. -
Peter Wippermann over 5 yearsWould you please elaborate how your answer solves the OP problem?