Hide soft keyboard on pressing back
Solution 1
Try this ...
create class called Util and put this code
public static void hideSoftKeyboard(Activity activity) {
final InputMethodManager inputMethodManager = (InputMethodManager) activity.getSystemService(Activity.INPUT_METHOD_SERVICE);
if (inputMethodManager.isActive()) {
if (activity.getCurrentFocus() != null) {
inputMethodManager.hideSoftInputFromWindow(activity.getCurrentFocus().getWindowToken(), 0);
}
}
}
and call on the onBackPressed() of Activity
Solution 2
I add below code in my BaseActivity.java
@Override
protected void onPause() {
super.onPause();
final InputMethodManager inputMethodManager = (InputMethodManager) getSystemService(Activity.INPUT_METHOD_SERVICE);
if (inputMethodManager != null && inputMethodManager.isActive()) {
if (getCurrentFocus() != null) {
inputMethodManager.hideSoftInputFromWindow(getCurrentFocus().getWindowToken(), 0);
}
}
}
Solution 3
Try like this,
@Override
public boolean onKeyDown(int keyCode, KeyEvent event) {
if (event.getAction() == KeyEvent.ACTION_DOWN) {
switch (keyCode) {
case KeyEvent.KEYCODE_BACK:
//useful for hiding the soft-keyboard is:
getWindow().setSoftInputMode(WindowManager.LayoutParams.SOFT_INPUT_STATE_ALWAYS_HIDDEN);
return true;
}
}
return super.onKeyDown(keyCode, event);
}
This may helps you
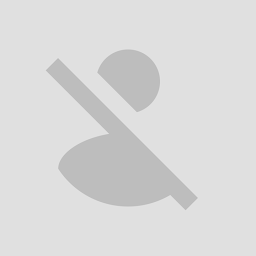
Amit Tiwari
Updated on June 30, 2022Comments
-
Amit Tiwari almost 2 years
I have an
EditText
in anActivity
and I want it to be active and soft-keyboard be open when I open thatActivity
. Here is myxml
forEditText
:<EditText android:background="@null" android:cursorVisible="true" android:elegantTextHeight="true" android:enabled="true" android:focusable="true" android:hint="Search" android:id="@+id/editText11" android:inputType="textNoSuggestions|textCapSentences" android:layout_centerVertical="true" android:layout_height="wrap_content" android:layout_width="match_parent" android:singleLine="true" android:textColor="#000000" android:textCursorDrawable="@null" />
and I have used
android:windowSoftInputMode="stateVisible"
for the activity in which I have thisEditText
.The problem is, when I press
back
once, the keyboard does not hide(ideally it does in all otherEditText
s) and when I pressback
again, it closes theActivity
. On the firstback
press, I am not getting a call toonBackPressed()
while on the secondback
press, I do. Why is this kind of behaviour is happening and how to resolve it?Edit What I want is, if keyboard is open, pressing back should close the keyboard and if the keyboard is not open, then close the activity.