How a meteor application knows if it's running on development, test or production environment?
Solution 1
Since Meteor 1.3 these flags work out of the box:
Meteor.isDevelopment
Meteor.isProduction
Meteor.isTest
Meteor.isAppTest
Solution 2
On the server:
var inDevelopment = function () {
return process.env.NODE_ENV === "development";
};
var inProduction = function () {
return process.env.NODE_ENV === "production";
};
Meteor sets the environment variable NODE_ENV to "development" when you run meteor
. In production, you can set the variable to whatever you want, otherwise it will default to "production".
Update: I created a smart package to allow this to work on the client and server.
mrt add allow-env
Just set permission rules in a server file.
allowEnv({
NODE_ENV: 1
});
Solution 3
You can use Meteor.settings
coupled with the --settings
option used when running meteor run
or meteor deploy
.
For example, to run in dev
mode, create a JSON file, call it meteorConfigDev.json, and put the following in it:
{
"public" : {
"mode" : "dev"
},
"anotherProperty" : "anotherValue"
}
Run your app using
meteor --settings meteorConfigDev.json
On the server and on the client you can access the "mode" using:
Meteor.settings.public.mode //in this case it will be "dev"
Note that settings in "public" are available on both the server and the client whereas everything else (in this case "anotherProperty") is only available on the server.
You can then have different configuration files for your different environments.
Solution 4
Very easy. I am running my app on five (yes, five!) different environments. I simply use a switch statement on the ROOT_URL as shown below for four different environments. Of course, you can use an if-else if you only have two environments. Works on the server. Just make a new file called startup.js and use the code example below. Cheers!
switch (process.env.ROOT_URL) {
case "http://www.production.com/":
BLOCK OF CODE HERE
break;
case "http://www.staging.com/":
BLOCK OF CODE HERE
break;
case "http://www.development.com/":
BLOCK OF CODE HERE
break;
case "http://localhost:3000/":
BLOCK OF CODE HERE
break;
}
In general, the format for a switch statement in javascript is
switch(expression) {
case n:
code block
break;
case n:
code block
break;
default:
default code block
}
UPDATE: Note that Meteor now provides Meteor.absoluteUrl()
, which is similar to process.env.ROOT_URL
with the addition of extra functionality. See docs.
Solution 5
There is an open pull request at github which would allow for that. Comment/Vote for it, so it is more likely to get included!
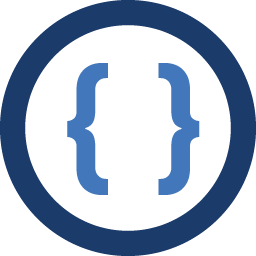
Admin
Updated on June 25, 2022Comments
-
Admin almost 2 years
I need to use different accounts provider's configurations when the meteor application runs as Development, Test or Production environment.
-
Corban Brook almost 12 yearsThis is only suitable for the most basic situations and will not work if you want to test your env on your local machine without doing a full deploy.
-
Mike Bannister almost 12 yearsYeah, definitely a stop gap. Works in the basic situations though. I use it to set the api id appropriately for the new auth system but I'm eager to replace it with a better solution.
-
DerWOK over 8 yearsThis does not work for me, as my app is self-hosted and when I launch my app via "meteor run --production" meteor still sets process.env.NODE_ENV to "development" (seems not reasonalbe, but they have their resons - see link). This is a known issue for years and e.g. discussed here: github.com/meteor/meteor/issues/1858.
-
opyh over 8 yearsI have seen several apps breaking because of similar code. This code makes your functionality dependent on a property that is independent from the environment (the URL). If the URL is changed, the functionality can break with potentially nobody noticing. Also you have to change one place more in your code now if you want to change the URL (or add another one), which you might have forgotten in a few months. Functionalities that are dependent on your environment are often vital for your app, not easy to test cleanly and not so well looked after, so you have to be extra careful.
-
Tamara Wijsman over 8 years@opyh: "How does X know if runs on Y?" is a question which questions the environment. How are you willing to answer that without inspecting the environment? Such answers would be out of scope. This has never broken in my experience; so, it seems you will need to come up with some examples and a better solution if you're so convinced this is wrong.
-
opyh over 8 yearsI did not advise against inspecting your
env
, but that you should not infer your environment from a URL, because the URL can change independently from your environment for various reasons. If your fellow coworker doesn't know that your ROOT_URL has a 'magic' extra-meaning for your app logic, there are 3 realistic cases that would break your app on production: 1) removewww.
, 2) changehttp
tohttps
, 3) have multiple URLs that belong to your production app and change the default URL. Also it is against the DRY principle. -
opyh over 8 yearsA better solution: Put your environment name in an extra
env
variable (e.g.process.env.METEOR_ENVIRONMENT
) or in the configuration, like go-oleg did. -
mc9 about 8 years"otherwise it will default to "production": doesn't it depend on where you host/what tools you use to host?