How can I access NTP clock in java
Solution 1
If want to access NTP info in Java you can create UDP packet conforming to the NTP packet format (NTP RFC-1305) setting mode field to MODE_CLIENT (3) then send the packet to NTP server on port 123 and listen for a response.
The Apache Commons Net library already has the framework to do this using only a few lines of code.
NTPUDPClient client = new NTPUDPClient();
client.open();
InetAddress hostAddr = InetAddress.getByName("*insert-target-server-host-name.com*");
TimeInfo info = client.getTime(hostAddr);
info.computeDetails(); // compute offset/delay if not already done
Long offsetValue = info.getOffset();
Long delayValue = info.getDelay();
String delay = (delayValue == null) ? "N/A" : delayValue.toString();
String offset = (offsetValue == null) ? "N/A" : offsetValue.toString();
System.out.println(" Roundtrip delay(ms)=" + delay
+ ", clock offset(ms)=" + offset); // offset in ms
client.close();
Note that the local clock offset (or time drift) is calculated with respect to the local clock and the NTP server's clock according to this standard NTP equation.
LocalClockOffset = ((ReceiveTimestamp - OriginateTimestamp) +
(TransmitTimestamp - DestinationTimestamp)) / 2
Where OriginateTimestamp (t1) is the local time the client sent the packet, ReceiveTimestamp(t2) is time request received by NTP server, TransmitTimestamp (t3) is time reply sent by server, and DestinationTimestamp (t4) is time at which reply received by client on local machine.
See client example for full code:
https://commons.apache.org/proper/commons-net/examples/ntp/NTPClient.java
Solution 2
When you setup ntp, System time will be synchronized with the ntp server time. When you use System.currentTimeMillis() will have value of the automatic adjusted system clock.
You should be aware that Timer can be sensitive to changes in the system clock, ScheduledThreadPoolExecutor isn't. You might check Scheduler Impacts with clock changes
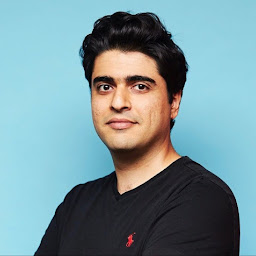
Mohammad Roohitavaf
Updated on June 05, 2022Comments
-
Mohammad Roohitavaf almost 2 years
I have a distributed program written in java. I want my nodes access a synchronized physical clock.
I know NTP is a protocol for physical clock synchronization. I know that I can install it on linux by sudo apt-get ntp.
My question is when I install it, how can I access this synchronized clock in my java program? I mean what happens when I install ntp on my machine? my system clock will be sync?
Thanks :)