How can I add class to the first and last item among the visible items of Owl Carousel 2?
Solution 1
DEMO:
http://plnkr.co/edit/t9URfKq9Mwh9jO705h7u?p=preview
Owl carousel adds a class active to all the visible items. So as you can see I have written a script below to loop through all the owl-item with class active and then using the 0th and last index, I am adding two different classes.
Use the code in your project and you will get the classes added.
jQuery(document).ready(function($) {
var carousel = $(".latest-work-carousel");
carousel.owlCarousel({
loop : true,
items : 3,
margin:0,
nav : true,
dots : false,
});
checkClasses();
carousel.on('translated.owl.carousel', function(event) {
checkClasses();
});
function checkClasses(){
var total = $('.latest-work-carousel .owl-stage .owl-item.active').length;
$('.latest-work-carousel .owl-stage .owl-item').removeClass('firstActiveItem lastActiveItem');
$('.latest-work-carousel .owl-stage .owl-item.active').each(function(index){
if (index === 0) {
// this is the first one
$(this).addClass('firstActiveItem');
}
if (index === total - 1 && total>1) {
// this is the last one
$(this).addClass('lastActiveItem');
}
});
}
});
DEMO:
http://plnkr.co/edit/t9URfKq9Mwh9jO705h7u?p=preview
Solution 2
I also had the same issue with styling the last active item and couldn't find anywhere for a easy fix. Finally came up with a fix that works for me.
Here's a simple fix that worked for me.
$('.owl-carousel')
.on('changed.owl.carousel initialized.owl.carousel', function(event) {
$(event.target)
.find('.owl-item').removeClass('last')
.eq(event.item.index + event.page.size - 1).addClass('last');
}).owlCarousel({
responsive: {
0: {
items: 3,
},
768: {
items: 4,
},
}
});
First item can be easy styled just using css.
.owl-item:not(.active) + .owl-item.active{
background: red;
}
Solution 3
Following @Lasithds answer, if you want to add "active" class to the first owl active div, you can use the following:
$('.owl-carousel').on('changed.owl.carousel initialized.owl.carousel', function (event) {
$(event.target)
.find('.owl-item').removeClass('first')
.eq(event.item.index).addClass('first');
})
.owlCarousel({
loop: false,
dots: false,
});
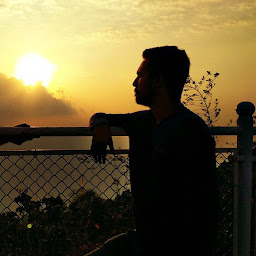
Shekhar R
Updated on June 16, 2022Comments
-
Shekhar R almost 2 years
I need to add a class to the first and last item of the currently visible items on Owl Carousel 2.
-
Shekhar R over 7 yearscan you provide a jsfiddle demo?
-
Mark Wilson over 7 yearsI have made a demo.
-
Shekhar R over 7 yearsyour code works fine but in my case, i want it to remain on the first and last active items even after scrolling the slider. like when you click on the next button, the first and last item changes right? i need our classes still to be on the visible items.
-
Mark Wilson over 7 yearsI was expecting this question. I will update the answer and demo.
-
Mark Wilson over 7 yearsI have updated the answer and demo. Please have a look.
-
lalit bhakuni about 6 yearsthis function only work on
single slider
it's possible thisfunction work
multiple slider -
Jacco van der Post almost 3 yearsThe JS doesn't work for me, selects the first active item instead of the last.