How can I add shadow to ImageButton?
may help you : You can use a Button:
<ImageButton
android:id="@+id/fab"
android:background="@drawable/ripple"
android:stateListAnimator="@anim/anim"
android:src="@drawable/ic_action_add"
android:elevation="4dp"
/>
where the ic_action_add
is your icon.
drawable/ripple.xml is:
<ripple xmlns:android="http://schemas.android.com/apk/res/android" android:color="?android:colorControlHighlight">
<item>
<shape android:shape="oval">
<solid android:color="?android:colorAccent" />
</shape>
</item>
</ripple>
anim/anim.xml is:
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:state_enabled="true"
android:state_pressed="true">
<objectAnimator
android:duration="@android:integer/config_shortAnimTime"
android:propertyName="translationZ"
android:valueFrom="@dimen/button_elevation"
android:valueTo="@dimen/button_press_elevation"
android:valueType="floatType" />
</item>
<item>
<objectAnimator
android:duration="@android:integer/config_shortAnimTime"
android:propertyName="translationZ"
android:valueFrom="@dimen/button_press_elevation"
android:valueTo="@dimen/button_elevation"
android:valueType="floatType" />
</item>
</selector>
Dimens.xml is :
<resources>
<dimen name="fab_size">56dp</dimen>
<dimen name="button_elevation">2dp</dimen>
<dimen name="button_press_elevation">4dp</dimen>
With the elevation attribute you should set the Outline via code:
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layoutfab);
//Outline
int size = getResources().getDimensionPixelSize(R.dimen.fab_size);
Outline outline = new Outline();
outline.setOval(0, 0, size, size);
findViewById(R.id.fab).setOutline(outline);
}
}
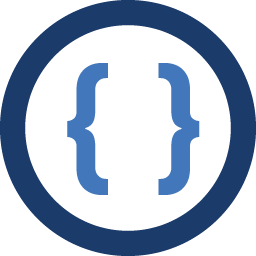
Admin
Updated on June 19, 2022Comments
-
Admin almost 2 years
this is my first time posting. And I've been using this website as a great resource. But I have come upon an issue. I've tried applying a shadow effect to my ImageButton but it doest work? What I'm trying to achieve is the shadow you can see on the floating bubble (Material Design). I have a circle ImageButton, but I wish to add a shadow around it. How can I achieve that?
This is circle_button.xml
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="oval"> <solid android:color="#C62666"/> </shape>
This is circle_button_pressed.xml
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="oval"> <solid android:color="#E92D6C"/> </shape>
This is my selector which combines both drawable's into one. button.xml
<?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android"> <item android:drawable="@drawable/circle_button" android:state_focused="true" android:state_pressed="true" /> <item android:drawable="@drawable/circle_button_pressed" android:state_focused="false" android:state_pressed="true" /> <item android:drawable="@drawable/circle_button_pressed" android:state_focused="true" /> <item android:drawable="@drawable/circle_button" android:state_focused="false" android:state_pressed="false" /> </selector>
And this is how I apply it to my ImageButton
<ImageButton android:id="@+id/btn_apply" android:layout_width="68dp" android:layout_height="68dp" android:layout_alignParentTop="true" android:layout_alignRight="@+id/scrollView1" android:layout_marginRight="45dp" android:layout_marginTop="94dp" android:adjustViewBounds="true" android:background="@drawable/button" android:scaleType="fitCenter" android:src="@drawable/apply_two" />
So again? How can I add a shadow to my button? Thanks for your time.