How can I call len() on an interface?
JSON parsing with maps in Go uses interfaces everywhere. Imagine you have the following JSON object:
{
"stuff" : [
"stuff1",
"stuff2",
"stuff3",
]
}
The Go JSON library will parse the outer object as a map from keys to values, as you've seen in your code. It maps variable names as keys to the values that correspond to those variable names. However, since it has no way of knowing ahead of time what those values are, the value type of the map is simply interface{}
. So let's say you know there's a key called "stuff"
, and you know that its value is an array. You could do:
arr := myMap["stuff"]
And you know that it's an array type, so you can actually instead do:
arr := myMap["stuff"].([]interface{})
the problem here is that while you're right that it's an array, and the JSON library knows this, it has no way of knowing that every element will be of type string
, so there's no way for it to decide that the array type should actually be []string
. Imagine if you had done this instead:
{
"stuff" : [
"stuff1",
"stuff2",
3
]
}
Well "stuff"
can't now be an array of strings because one of the elements isn't a string. In fact, it can't be an array of anything - there's no single type that would satisfy the types of all of the elements. So that's why the Go JSON library has no choice but to leave it as []interface{}
. Luckily, since all you want is the length, you're already done. You can just do:
arr := myMap["stuff"].([]interface{})
l := len(arr)
Now that's all fine and good, but let's say that down the road you want to actually look at one of the elements. You could now take out an element and, knowing that it's a string, do:
arr := myMap["stuff"].([]interface{})
iv := arr[0] // interface value
sv := iv.(string) // string value
NOTE
When I say "array," I mean array in the JSON sense - these are JSON arrays. The data structure that represents them in Go is called a "slice" (Go has arrays too, but they're a separate thing - if you're used to arrays in languages like C or Java, Go slices are the closest analogue).
Related videos on Youtube
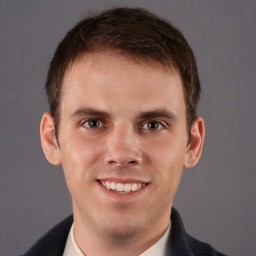
Kevin Burke
I build reliable software and design great experiences. I'm available for hire: https://burke.services
Updated on September 14, 2022Comments
-
Kevin Burke 9 months
I'm writing a test that a JSON list is empty.
{"matches": []}
The object has type
map[string]interface{}
, and I want to test that the list is empty.var matches := response["matches"] if len(matches) != 0 { t.Errorf("Non-empty match list!") }
However I'm told at compile time that this is invalid
invalid argument matches (type interface {}) for len
If I try casting to a list type:
matches := response["matches"].([]string)
I get a panic:
panic: interface conversion: interface is []interface {}, not []string [recovered]
What do I want to write here?
-
Dustin over 9 yearsIf you can avoid it, don't use
interface{}
. It isn't necessary music of the time. e.g., parse that as `map[string][]string if that's what you want. Or a struct
-
-
Tyler over 9 yearsForgive me for nitpicking, but
[]interface{}
is not an array (in Go terminology), it's a slice. -
joshlf over 9 yearsI know - I meant array in the JSON sense.