How can I check valid format of Aadhar card, driving license and Pan Card in Flutter programmatically
303
The fastest and easiest solution is to use a regex expression:
extension AadharNumberValidator on String {
bool isValidAadharNumber() {
return RegExp(
r'^[2-9]{1}[0-9]{3}\\s[0-9]{4}\\s[0-9]{4}$')
.hasMatch(this);
}
}
extension PanCardValidator on String {
bool isValidPanCardNo() {
return RegExp(
r'^[A-Z]{5}[0-9]{4}[A-Z]{1}$')
.hasMatch(this);
}
}
extension DrivingLicense Validator on String {
bool isValidLicenseNo() {
return RegExp(
r'^(([A-Z]{2}[0-9]{2})( )|([A-Z]{2}-[0-9]{2}))((19|20)[0-9][0-9])[0-9]{7}$')
.hasMatch(this);
}
}
And use an extension of String for validate
TextFormField(
autovalidate: true,
validator: (input) => input.isValidAadharNumber() ? null : "Check your aadhar number",
)
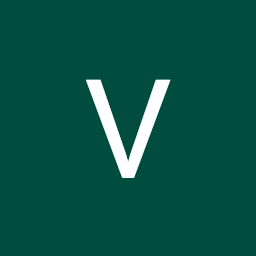
Author by
Vrusti Patel
Updated on November 23, 2022Comments
-
Vrusti Patel over 1 year
How can I check the validity of the format of a Aadharcard, driving licence and Pan Card in the textform field in Flutter programmatically?
-
Admin over 2 yearsYour answer could be improved with additional supporting information. Please edit to add further details, such as citations or documentation, so that others can confirm that your answer is correct. You can find more information on how to write good answers in the help center.