How can I convert a json string to a scala map?
Solution 1
I tried the following method with json4s
3.2.11 and it works:
import org.json4s._
import org.json4s.jackson.JsonMethods._
//...
def jsonStrToMap(jsonStr: String): Map[String, Any] = {
implicit val formats = org.json4s.DefaultFormats
parse(jsonStr).extract[Map[String, Any]]
}
Maybe you didn't define the implicit val
of type Formats
? Note also that you don't need to have an implicit val
within every and each method as long as it's findable in the scope.
Solution 2
You can use the following code to parse a JSON string into a Map[String, Any]
val jsonMap = parse(jsonString).values.asInstanceOf[Map[String, Any]]
However, this is not typesafe and hence should be used with caution when extracting values from the map.
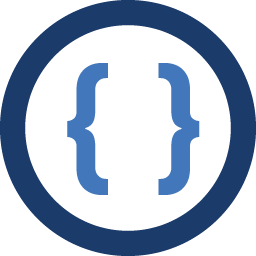
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I have a nested json whose structure is not defined. It can be different each time I run since I am reading from a remote file. I need to convert this json into a map of type
Map[String, Any]
. I tried to look into json4s and jackson parsers but they don't seem to solve this issue I have. Does anyone know how I can achieve this?Example string:
{"body":{ "method":"string", "events":"string", "clients":"string", "parameter":"string", "channel":"string", "metadata":{ "meta1":"string", "meta2":"string", "meta3":"string" } }, "timestamp":"string"}
The level of nesting can be arbitrary and not predefined.
To help with the use case:
I have a Map[String,Any] which I need to store in a file as backup. So I convert it to a json string and store it in a file. Now everytime I get new data, I need to get the json from the file, convert it to a map again and perform some computation. I cannot store the map in memory since I would lose that if my job fails.
I need a solution that would convert the json string back to the original map I had before i converted it.