How can I create a custom form in WordPress?
To answer question one: WordPress provides action and filter hooks for developers to add their custom PHP code or functions. You should look into that, because using plugins that produce snippets will not help in your case, because it causes your PHP code to execute without even loading your form, so you will be seeing your success page instead.
To learn more about action and filter hooks visit here.
Alternatively to us action/filter hooks, you can upload your PHP file into the theme folder. However, there's a flaw to that. Your file may be lost when WordPress updates.
To answer question two: There is an easier way to validate your form if using JavaScript. All you need to do is add the word 'required' within the input tag. You can also use input type 'email' together with the required keyword to validate you email. See the example below.
<form name="myForm" method="POST" action="../process.php">
Your Name: <input id="customer_name" name="customer_name" type="text" required/>
Your Email: <input id="customer_email" name="customer_email" type="email" required/>
Sex: <input name="customer_sex" type="radio" value="male" />Male <input name="customer_sex" type="radio" value="female" />Female
Your Age: <input id="customer_age" name="customer_age" type="text" />
<input type="submit" value="Submit" />
</form>
If you still want to use your JavaScript function, try using document.getElementById('customer_name')
and document.getElementById('customer_email')
instead of document.forms
. Also make sure you close your script tag at the end. See below for an example.
<script type="text/javascript">
function form_validation() {
/* Check the Customer Name for blank submission */
var customer_name = document.getElementById('customer_name').value;
if (customer_name == "" || customer_name == null) {
alert("Name field must be filled.");
return false;
}
/* Check the Customer Email for invalid format */
var customer_email = document.getElementById('customer_email').value;
var at_position = customer_email.indexOf("@");
var dot_position = customer_email.lastIndexOf(".");
if (at_position < 1 ||
dot_position < at_position + 2 ||
dot_position + 2 >= customer_email.length) {
alert("Given email address is not valid.");
return false;
}
}
</script>
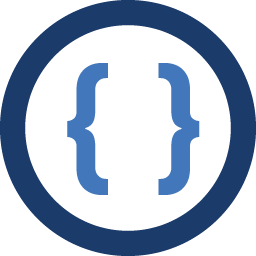
Admin
Updated on July 19, 2022Comments
-
Admin almost 2 years
I have a WordPress site and would like to create a form (input → database).
I've seen two tutorials:
- How you can easily create customized form in WordPress
- How to create custom forms in WordPress without using plugins?
They're both very similar. Creating the front end with HTML and JavaScript, and then processing the information to the database using PHP.
My problem is that whenever I submit the form, it shows a 404 page which says:
Oops! That page can’t be found.
Now my problem is (or I want to know):
-
Where do I need to put the
process.php
file? (I'm using FileZilla). I've tried several places in thepublic_html/wp-content
folder. -
Why aren't the name and email fields being validated? (no alert for empty name field, etc.)
Form structure:
Your Name: [TEXT], Your Email: [TEXT], Sex: [*male *female], Your Age:[TEXT], [Submit Button]
Form page:
<form name="myForm" method="POST" onsubmit="return form_validation()" action="../process.php"> Your Name: <input id="customer_name" name="customer_name" type="text" /> Your Email: <input id="customer_email" name="customer_email" type="text" /> Sex: <input name="customer_sex" type="radio" value="male" />Male <input name="customer_sex" type="radio" value="female" />Female Your Age: <input id="customer_age" name="customer_age" type="text" /> <input type="submit" value="Submit" /> </form> <script type="text/javascript"> function form_validation() { /* Check the Customer Name for blank submission */ var customer_name = document.forms["myForm"]["customer_name"].value; if (customer_name == "" || customer_name == null) { alert("Name field must be filled."); return false; } /* Check the Customer Email for invalid format */ var customer_email = document.forms["myForm"]["customer_email"].value; var at_position = customer_email.indexOf("@"); var dot_position = customer_email.lastIndexOf("."); if (at_position < 1 || dot_position < at_position + 2 || dot_position + 2 >= customer_email.length) { alert("Given email address is not valid."); return false; } } </script>
File
process.php
(not edited):<?php $customer_name = $_POST["customer_name"]; $customer_email = $_POST["customer_email"]; $customer_sex = $_POST["customer_sex"]; $customer_age = $_POST["customer_age"]; $conn = mysqli_connect("Database Host", "Database Username", "Database Password", "Database Name"); if(!$conn) { die(‘Problem in database connection: ‘ . mysql_error()); } $query = "INSERT INTO ‘Database Name’.’Table Name’ ( ‘customer_name’, ‘customer_email’, ‘customer_sex’, ‘customer_age’ ) VALUES ( $customer_name, $customer_email, $customer_sex, $customer_age )"; mysqli_query($conn, $query); header("Location: my-site.com/success"); // Redirects to success page ?>
-
Peter Mortensen over 4 yearsThe link is (effectively) broken: "Plugin API/Action Reference. There is currently no text in this page."